PHP Tutorial Laravel 4 Framework (Authentication) - Activating User Account II - Update user's status
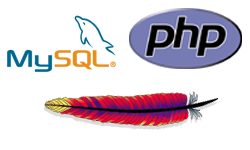
Laravel 4
- Installing on Ubuntu - local
- Installing on a Shared host
- Installing on Windows
- Creating users table
- Home page with controller and blade
- Blade Templating
- Database connection and sending emails
- Creating user account I - GET
- Creating user account II - POST
- Creating user account III - Error checking & redirecting input
- Creating user account IV - User::create()
- Activating user account I - Mail::send()
- Activating user account II - Update user's status
- User account sign-in I - Route and link
- User account sign-in II - Validation
- User account sign-in III - Login Authentication
- Singing out
- Remember the user
- Changing Password I
- Changing Password II
- Recovering forgotten password I
- Recovering forgotten password II
- User Profile
- Database Migration using artisan
This tutorial is the continuation from Activating user account I - Mail::send().
Now that the user can receive an account activation email, we want to process the email reply from the user so that the user can login.
In this section, we'll get information about the user whose database hash code is the same as the code in the reply email for the activation.
AccountController.php file:
<?php class AccountController extends BaseController { /* Viewing the form */ public function getCreate() { return View::make('account.create'); } /* Submitting the form */ public function postCreate() { $validator = Validator::make(Input::all(), array( 'email' => 'required|max:50|email|unique:users', 'username' => 'required|max:20|min:3|unique:users', 'password' => 'required|min:6', 'password_again'=> 'required|same:password' ) ); if($validator->fails()) { return Redirect::route('account-create') ->withErrors($validator) ->withInput(); // fills the field with the old inputs what were correct } else { // create an account $email = Input::get('email'); $username = Input::get('username'); $password = Input::get('password'); // Activation code $code = str_random(60); /* This does the same as User::create() $user = new User; $user->fill(array( 'email' => $email, 'username' => $username, 'password' => Hash::make($password), 'code' => $code, 'active' => 0, )); $userdata = $user->save(); */ // record $userdata = User::create(array( 'email' => $email, 'username' => $username, 'password' => Hash::make($password), 'code' => $code, 'active' => 0 )); if($userdata) { Mail::send('emails.auth.activate', array('link' => URL::route('account-activate', $code), 'username' => $username), function($message) use ($userdata) { $message->to($userdata->email, $userdata->username)->subject('Activate your account'); }); return Redirect::route('home') ->with('global', 'Your account has been created. We have sent you an email to activate your accout'); } } } public function getActivate($code) { $user = User::where('code', '=', $code)->where('active', '=', 0); /* if user is available */ if($user->count()) { $user = $user->first(); // Update the user status to active $user->active = 1; $user->code = ''; if($user->save()) { return Redirect::route('home') ->with('global', 'Activated! You can now sign in!'); } } /* fall back */ return Redirect::route('home') ->with('global', 'We could not activate your account. Try again later.'); } }
When the user click the link, and if it's not successful (hash code not matching, for example, like this uri, http://localhost/account/activate/abc ):
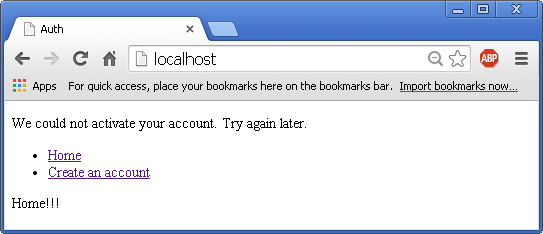
But when successful:
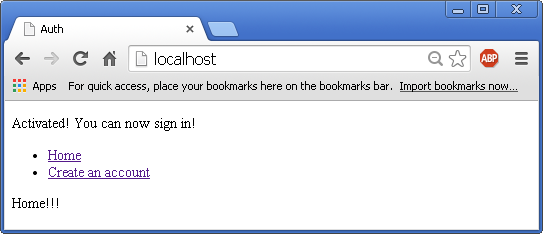
After the successful operation, the user's state has been switched to 1 from 0 and the code is now empty string:
Before:

==>
After;

Laravel 4
- Installing on Ubuntu - local
- Installing on a Shared host
- Installing on Windows
- Creating users table
- Home page with controller and blade
- Blade Templating
- Database connection and sending emails
- Creating user account I - GET
- Creating user account II - POST
- Creating user account III - Error checking & redirecting input
- Creating user account IV - User::create()
- Activating user account I - Mail::send()
- Activating user account II - Update user's status
- User account sign-in I - Route and link
- User account sign-in II - Validation
- User account sign-in III - Login Authentication
- Singing out
- Remember the user
- Changing Password I
- Changing Password II
- Recovering forgotten password I
- Recovering forgotten password II
- User Profile
- Database Migration using artisan
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization