PHP Tutorial Laravel 4 Framework (Authentication) - Recovering Forgotten Password II
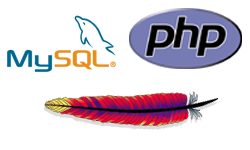
Laravel 4
- Installing on Ubuntu - local
- Installing on a Shared host
- Installing on Windows
- Creating users table
- Home page with controller and blade
- Blade Templating
- Database connection and sending emails
- Creating user account I - GET
- Creating user account II - POST
- Creating user account III - Error checking & redirecting input
- Creating user account IV - User::create()
- Activating user account I - Mail::send()
- Activating user account II - Update user's status
- User account sign-in I - Route and link
- User account sign-in II - Validation
- User account sign-in III - Login Authentication
- Singing out
- Remember the user
- Changing Password I
- Changing Password II
- Recovering forgotten password I
- Recovering forgotten password II
- User Profile
- Database Migration using artisan
This tutorial is the continuation from Recovering forgotten password I.
In previous chapter, we saw our code passed validation step. Now, we'll continue to work on how we can recover user's forgotten password.
We need to write a code after passing the validation. Let's open AccountController.phpfile, modify it like this:
public function postForgotPassword() { $validator = Validator::make(Input::all(), array( 'email' => 'required|email' ) ); if($validator->fails()) { return Redirect::route('account-forgot-password') ->withErrors($validator) ->withInput(); } else { $user = User::where('email', '=', Input::get('email')); if($user->count()) { // Generate a new code and password $code = str_random(60); $password = str_random(10); $user = $user->first(); $user->code = $code; $user->password_temp = Hash::make($password); if($user->save()) { die(); } } } /* fall back */ return Redirect::route('account-forgot-password') ->with('global', 'Could not request new password.'); }
What the code is doing:
- Grab the user
- Generate the code and password (hashed)
- Update our database: code and password_temp
- Save() and die()
Here is the data in our database for the user before we run the code:

After we run the code and after updating database:

We can see that the code successfully updated code and temporary password, password_temp after taking the correct user's email as an input for user's request for the password recover.
The code will be sent to the user and once confirmed, the password_temp will replace the old password. Then, the user will be able to sign in with the temporary password and can recover the forgotten password.
To send an email, we need a new view. So, let's open a new file, views/emails/auth/forgot.blade.php:
Hello {{ $username}}, You requested a new password. You'll need to use the following link to activate. New password: {{ $password }} <br><br> ---<br> {{ $link }}<br> ---<br>
Then, the code to send an email is in AccountController.php:
public function postForgotPassword() { $validator = Validator::make(Input::all(), array( 'email' => 'required|email' ) ); if($validator->fails()) { return Redirect::route('account-forgot-password') ->withErrors($validator) ->withInput(); } else { $user = User::where('email', '=', Input::get('email')); if($user->count()) { $user = $user->first(); // Generate a new code and password $code = str_random(60); $password = str_random(10); $user->code = $code; $user->password_temp = Hash::make($password); $username = $user->username; if($user->save()) { Mail::send('emails.auth.forgot', array('link'=>URL::route('account-recover', $code), 'username'=>$username, 'password'=>$password), function($message) use ($user){ $message->to($user->email, $use->username)->subject('Your new password'); }); return Redirect::route('home') ->with('global', 'We have sent you a new password by email'); } } } /* fall back */ return Redirect::route('account-forgot-password') ->with('global', 'Could not request new password.'); }
For the recover page with the code, we should add the following lines to routes.php:
Route::get('/account/recover/{code}', array('as' => 'account-recover', 'uses' => 'AccountController@getRecover' ));
Finally, the code passed in should be display in the recover page. So, we need to add the following lines to AccountController.php for the getRecover() method:
public function getRecover($code) { return $code; }
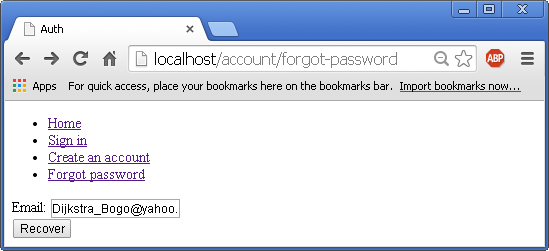
To recover forgotten password, we press "Recover". Then, as shown in the picture below, we'll get the message saying ".. sent you a password ...". Also note that we're redirected back to the 'home' page.
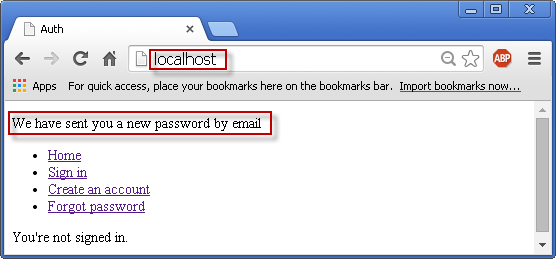
Here is the email received:
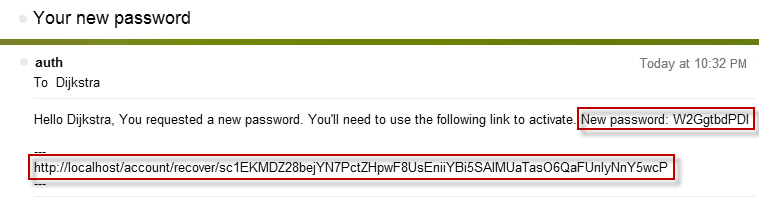
Can the user use the password sent via email and sign in?
No, not yet. That's because the password is not actual password in our database, but it is password_temp as shown in the code below:
$user->password_temp = Hash::make($password);
we haven't replaced old password with the new yet.
What for the code in the email?
We're going to use that to find the user with the same code in the database. But for now, if the user click the link, it will just be printed out doing nothing:
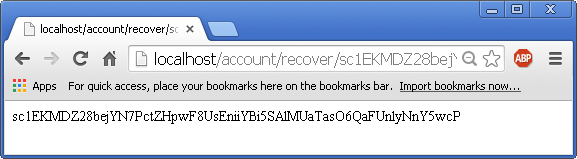
It's just doing what's been told to do:
public function getRecover($code) { return $code; }
Let's work on getRecover() method in AccountController:
public function getRecover($code) { $user = User::where('code', '=', $code) ->where('password_temp', '!=', ''); if($user->count()) { $user = $user->first(); $user->password = $user->password_temp; $user->password_temp = ''; $user->code = ''; if($user->save()) { return Redirect::route('home') ->with('global', 'Your account has been recovered and you can sign in with your password.'); } } return Redirect::route('home') ->with('global', 'Count not recover your account.'); }
If the user click the link in the email, we get this:
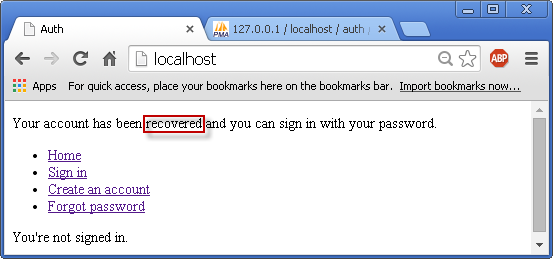
Also, we can check our database:

As we can see from the picture, we have a new password and the fields for code and password_temp are now empty strings.
Now the user can sign-in with the new password received via email!
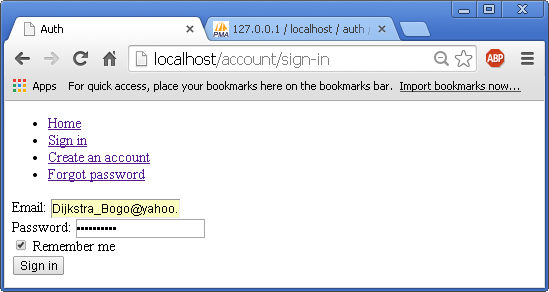
Signed In!
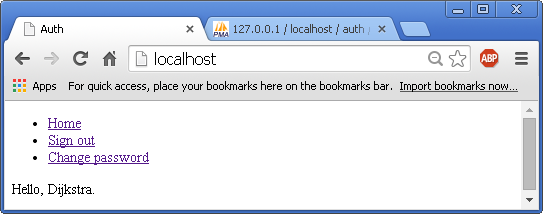
The final project file is here: auth.zip.
Laravel 4
- Installing on Ubuntu - local
- Installing on a Shared host
- Installing on Windows
- Creating users table
- Home page with controller and blade
- Blade Templating
- Database connection and sending emails
- Creating user account I - GET
- Creating user account II - POST
- Creating user account III - Error checking & redirecting input
- Creating user account IV - User::create()
- Activating user account I - Mail::send()
- Activating user account II - Update user's status
- User account sign-in I - Route and link
- User account sign-in II - Validation
- User account sign-in III - Login Authentication
- Singing out
- Remember the user
- Changing Password I
- Changing Password II
- Recovering forgotten password I
- Recovering forgotten password II
- User Profile
- Database Migration using artisan
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization