PHP & MySQL Tutorial
cURL
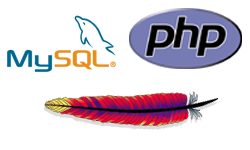
cURL is a command line tool for getting or sending files using URL syntax.
Since cURL uses libcurl, and PHP supports libcurl, we can use cURL to connect and communicate to many different types of servers with many different types of protocols. libcurl currently supports the http, https, ftp, gopher, telnet, dict, file, and ldap protocols. libcurl also supports HTTPS certificates, HTTP POST, HTTP PUT, FTP uploading (this can also be done with PHP's ftp extension), HTTP form based upload, proxies, cookies, and user+password authentication.
- curl_close - Close a cURL session
- curl_copy_handle - Copy a cURL handle along with all of its preferences
- curl_errno - Return the last error number
- curl_error - Return a string containing the last error for the current session
- curl_exec - Perform a cURL session
- curl_getinfo - Get information regarding a specific transfer
- curl_init - Initialize a cURL session
- curl_setopt_array - Set multiple options for a cURL transfer
- curl_setopt - Set an option for a cURL transfer
- curl_version - Gets cURL version information
The following example gets "mypic.mp4" file using ftp, and saving it in the current directory with the same name:
<?php $username = 'usrname'; $password = 'passwd'; $source_file ="mypic.mp4"; $url = "bogotobogo.com/public_html/php/files/".$source_file; $ftp_server = "ftp://" . $username . ":" . $password . "@" . $url; $target_file = $source_file; $out = fopen($target_file, 'wb'); echo "ftp_server=".$ftp_server."\n"; echo "Starting CURL.\n"; $ch = curl_init(); echo "Set CURL URL.\n"; curl_setopt($ch, CURLOPT_FILE, $out); curl_setopt($ch, CURLOPT_HEADER, 0); curl_setopt($ch, CURLOPT_FOLLOWLOCATION, 1); curl_setopt($ch, CURLOPT_TIMEOUT, 3600); curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 10); curl_setopt($ch, CURLOPT_USERAGENT, "Mozilla/5.0"); curl_setopt($ch, CURLOPT_URL, $ftp_server); set_time_limit(0); $ret = curl_exec($ch); set_time_limit(30); fclose($out); curl_close($ch); ?>
Output is:
ftp_server=ftp://usrname:passwd@bogotobogo.com/public_html/php/files/mypic.mp4 Starting CURL. Set CURL URL.
The options for curl_setopt are:
- CURLOPT_CONNECTTIMEOUT
The number of seconds to wait while trying to connect. Use 0 to wait indefinitely. - CURLOPT_FILE
The file that the transfer should be written to. The default is STDOUT (the browser window). - CURLOPT_FOLLOWLOCATION
TRUE to follow any "Location: " header that the server sends as part of the HTTP header (note this is recursive, PHP will follow as many "Location: " headers that it is sent, unless CURLOPT_MAXREDIRS is set). - CURLOPT_HEADER
TRUE to include the header in the output. - CURLOPT_RETURNTRANSFER
TRUE to return the transfer as a string of the return value of curl_exec() instead of outputting it out directly. - CURLOPT_TIMEOUT
The maximum number of seconds to allow cURL functions to execute. - CURLOPT_USERAGENT
The contents of the "User-Agent: " header to be used in a HTTP request. - CURLOPT_USERAGENT
The URL to fetch. This can also be set when initializing a session with curl_init().
This is an example for hitting url for ad_tracker.
<?php $url = "http://ad.doubleclick.net/ad/DZ982/;order=tiny1234;sz=1x1;ord=831434317"; echo "Starting CURL.\n"; $ch = curl_init(); echo "Set CURL URL.\n"; curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); curl_setopt($ch, CURLOPT_FOLLOWLOCATION, 1); curl_setopt($ch, CURLOPT_TIMEOUT, 10); curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 10); curl_setopt($ch, CURLOPT_USERAGENT, "Mozilla/5.0"); curl_setopt($ch, CURLOPT_URL, $url); echo "Set CURL exec\n"; $xml = curl_exec($ch); if(!curl_errno($ch)) { $info = curl_getinfo($ch); $ret = $info["http_code"]; } else { $ret = 400; } echo "ret=".$ret."\n"; echo "Set CURL close\n"; curl_close($ch); ?>
Output is:
Starting CURL. Set CURL URL. Set CURL exec ret=200 Set CURL close
Also see C++ url hitting using wget and tcpdump
- -I/--head
(HTTP/FTP) Fetch the HTTP-header only! HTTP-servers feature the command HEAD which this uses to get nothing but the header of a document. When used on a FTP file, curl displays the file size only. If this option is used twice, the second will again disable header only. - -A/--user-agent
(HTTP) Specify the User-Agent string to send to the HTTP server. Some badly done CGIs fail if its not set to "Mozilla/5.0". To encode blanks in the string, surround the string with single quote marks. This can also be set with the -H/--header flag of course. If this option is set more than once, the last one will be the one that's used.
$ curl -I -A Mozilla/5.0 http://ad.doubleclick.net/ad/DZ982/;order=tiny1234;sz=1x1;ord=[timestamp]? HTTP/1.1 302 Moved Temporarily Content-Length: 0 Date: Thu, 15 Dec 2012 02:17:51 GMT Location: http://mytest.net/myad/pixel.gif Cache-Control: no-cache Pragma: no-cache Set-Cookie: test_cookie=CheckForPermission; path=/; domain=.doubleclick.net; expires=Thu, 15 Dec 2012 02:32:51 GMT P3P: CP="CURa ADMa DEVa PSAo PSDo OUR BUS UNI PUR INT DEM STA PRE COM NAV OTC NOI DSP COR" Server: GFE/2.0 Content-Type: text/html
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization