PHP & MySQL Tutorial - Response to a request
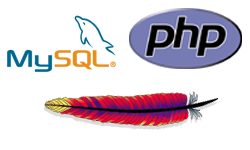
We had a signup form in the previous chapter:
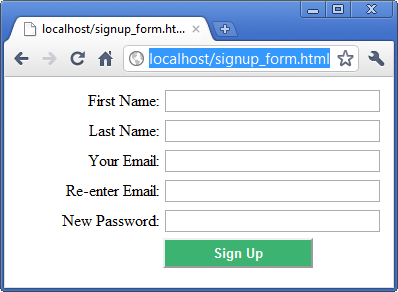
I put the signup form file into public_html under C:/server/www/apacheserver.dev/ directory.
We can use either localhost/signup_form.html (picture above) or
http://www.apacheserver.dev/signup_form.html (picture below) as our URL for browser.
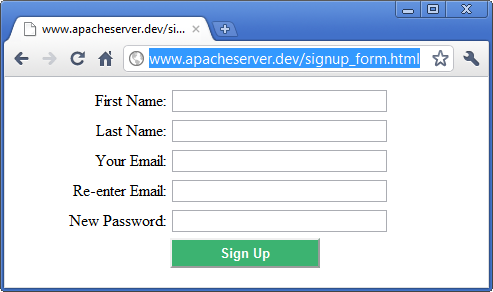
User typed information into the signup form as follows.
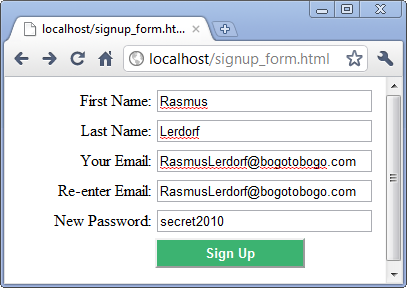
Here is the returned web page after processing the user input.
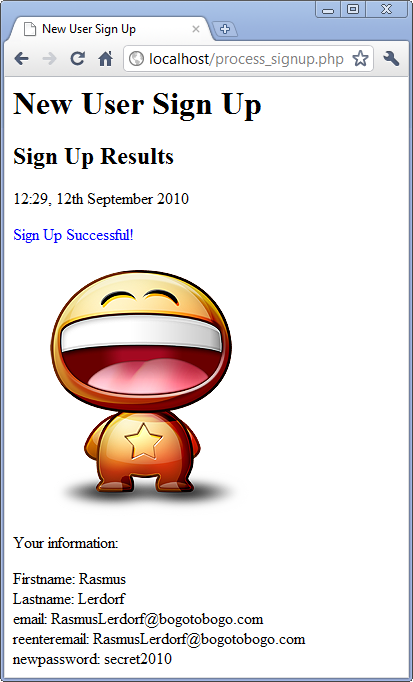
Let's look into how it's done.
The following statement creates a new variable named $firstname and copies the contents of $_POST['firstname'] into the new variable:
$firstname = $_POST['firstname'];
Same to other variables as we see below.
<html> <head> <title>New User Sign Up</title> </head> <body> <h1>New User Sign Up</h1> <h2>Sign Up Results</h2> <?php $firstname = $_POST['firstname']; $lastname = $_POST['lastname']; $email = $_POST['email']; $reenteremail = $_POST['reenteremail']; $newpassword = $_POST['newpassword']; date_default_timezone_set('America/Los_Angeles'); echo date('H:i, jS F Y'); echo '<p><font color="blue">Sign Up Successful!</font></p>'; echo '<img src="images/php1/happy_face.png" alt="happy face"/>'; echo '<p>Your information: </p>'; echo 'Firstname: '.$firstname.'<br />'; echo 'Lastname: '.$lastname.'<br />'; echo 'email: '.$email.'<br />'; echo 'reenteremail: '.$reenteremail.'<br />'; echo 'newpassword: '.$newpassword.'<br />'; ?> </body> </html>
In the code, the following lines create 5 variables:
$firstname, $lastname, $email, $reenteremail, and $newpassword and sets them to contain the data sent via the POST method from the form.
To make the scripts start doing something visible, we add the following lines to the bottom of our PHP script:
echo 'Firstname: '.$firstname.'<br />'; echo 'Lastname: '.$lastname.'<br />'; echo 'email: '.$email.'<br />'; echo 'reenteremail: '.$reenteremail.'<br />'; echo 'newpassword: '.$newpassword.'<br />';
Taking data from the user and sending produced output to the browser like this is a risky practice from a security perspective. We should filter input data. We'll go over this issue in later chapter.
In the example script, echo prints the value in each form field, preceded by some explanatory name, then HTML <br /> after.:
echo 'Firstname: '.$firstname.'<br />';
Notice we used period(.) between them.
This period is the string concatenation operator, which adds strings. We'll often use it when we send output to the browser with echo. This way, we can avoid writing multiple echo command.
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization