PHP Tutorial Laravel 4 Framework (Authentication) - Creating User Account I
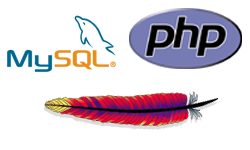
Laravel 4
- Installing on Ubuntu - local
- Installing on a Shared host
- Installing on Windows
- Creating users table
- Home page with controller and blade
- Blade Templating
- Database connection and sending emails
- Creating user account I - GET
- Creating user account II - POST
- Creating user account III - Error checking & redirecting input
- Creating user account IV - User::create()
- Activating user account I - Mail::send()
- Activating user account II - Update user's status
- User account sign-in I - Route and link
- User account sign-in II - Validation
- User account sign-in III - Login Authentication
- Singing out
- Remember the user
- Changing Password I
- Changing Password II
- Recovering forgotten password I
- Recovering forgotten password II
- User Profile
- Database Migration using artisan
This tutorial is the continuation from Database connection and sending emails.
In this chapter, we'll write a code to check if the user is a guest or not. Also, we will have a code to prevent Cross-site request forgery (CSRF)
We need to change our DocumentRoot to C:\Apache2\htdocs\bogotobogo\Laravel\auth\public from C:\Apache2\htdocs. Otherwise, we may get the following error when we make uri request:
public/account/create was not found on this server.
We may avoid the issue by using url like this:
public/index.php/account/create
But by switching the DocumentRoot we can use clean url.
To set virtual host, we need to modify the following files.
- C:\Apache2\conf\httpd.conf
# Virtual hosts Include conf/extra/httpd-vhosts.conf
- C:\Apache2\conf\extra\httpd-vhosts.conf
<VirtualHost *:80> DocumentRoot "C:\Apache2\htdocs\bogotobogo\Laravel\auth\public" ServerName authentication.dev ServerAlias www.authentication.dev <directory "C:\Apache2\htdocs\bogotobogo\Laravel\auth\public"> AllowOverride All Options Indexes FollowSymLinks Order allow,deny Allow from all </directory> </VirtualHost>
- C:\WINDOWS\system32\drivers\etc\hosts
127.0.0.1 localhost 127.0.0.1 authentication.dev 127.0.0.1 www.authentication.dev
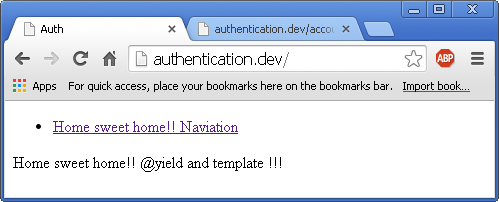
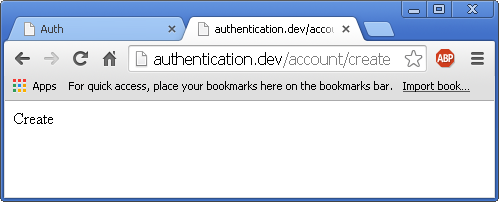
Route filters provide a convenient way of limiting access to a given route, which is useful for creating areas of our site which require authentication. There are several filters included in the Laravel framework, including an auth filter, an auth.basic filter, a guest filter, and a csrf filter. These are located in the app/filters.php file.
routes.php:
<?php Route::get('/', array('as' => 'home', 'uses' => 'HomeController@home' )); /* Unauthenticated group */ Route::group(array('before' => 'guest'), function() { /* CSRF protection */ Route::group(array('before' => 'csrf'), function() { }); /* Create an account (GET) */ Route::get('/account/create', array('as' => 'account-create', 'uses' => 'AccountController@getCreate' )); });
In the code, we used 'before' => 'guest' because we don't want an already logged in user to be able to login again. Laravel comes with the default 'guest filter (see app/filters.php).
For CSRF Protection, please check http://laravel.com/docs/security.
views/account/create.blade.php:
view from views.account.create (create.blade.php)
controller/AccountController.php:
<?php class AccountController extends BaseController { /* Viewing the form */ public function getCreate() { return View::make('account.create'); } /* Submitting the form */ public function postCreate() { } }
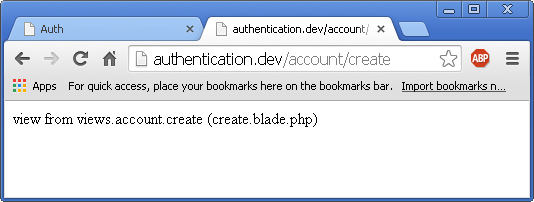
Laravel 4
- Installing on Ubuntu - local
- Installing on a Shared host
- Installing on Windows
- Creating users table
- Home page with controller and blade
- Blade Templating
- Database connection and sending emails
- Creating user account I - GET
- Creating user account II - POST
- Creating user account III - Error checking & redirecting input
- Creating user account IV - User::create()
- Activating user account I - Mail::send()
- Activating user account II - Update user's status
- User account sign-in I - Route and link
- User account sign-in II - Validation
- User account sign-in III - Login Authentication
- Singing out
- Remember the user
- Changing Password I
- Changing Password II
- Recovering forgotten password I
- Recovering forgotten password II
- User Profile
- Database Migration using artisan
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization