PHP & MySQL Tutorial
Cookies and Sessions II
- Access Limit and Starting a Session
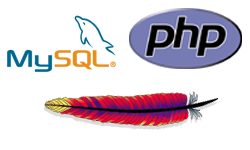
Cookies can be used to prevent direct access to pages of a website without first logging in to that site. After the log-in process creates the cookie, PHP scripts on all other pages check if there is the cookie before showing contents.
The example below, the log-in page creates a cookie called authorization with a value of ok when the user submits the form. The browser is redirected to the next page that looks for the cookie. If it is not able to find the cookie, the browser is redirected to the log-in page.
<?php if(isset($_POST['login_button'])) { $user = $_POST['username']; $pass = $_POST['password']; if( $user && $pass ) { setcookie("authorization","ok" ); header( "Location:loggedin.php"); exit(); } } ?> <html> <head> <title>Setting Cookie</title> </head> <body> <form method="post" action="<?php echo $_SERVER['PHP_SELF']; ?>" > Username: <input type="text" name="username"> <br /> Password: <input type="text" name="password"> <br /> <br /> <input type="submit" name="login_button" value="Log In"> </form> </body> </html>
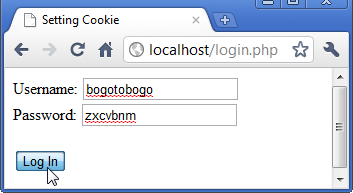
<?php $auth = $_COOKIE['authorization']; header ("Cache-Control:no-cache"); if(!$auth == "ok") { header ("Location:login.php"); exit(); } ?> <html> <head> <title>Logged In</title> </head> <body> <p>Successful log-in.</p> </form> </body> </html>
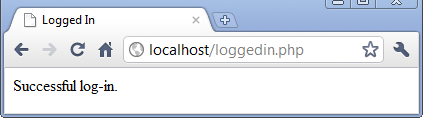
Another way to make data accessible across the various web pages is using a session. Sessions in PHP are driven by a unique session ID, a cryptographically random number. This session ID is generated by PHP and stored on the client side for the lifetime of a session. It can be either stored on a user's computer in a cookie or passed along through URLs.
The session ID acts as a key that allows us to register particular variables known as session variables. The contents of these variables are stored at the server. The session ID is the only information visible at the client side. So, if the session ID is visible either through a cookie or the URL at the time of a particular connection to our site, we can access the session variables stored on the server for that session. By default, the session variables are stored in flat files on the server.
So, when we see our URL contains a string of random-looking data, it is most likely to be one of the forms of session control.
Session creates a file in a temporary directory on the server where registered session variables, and their values, can be stored. This data will be available to all pages on the site during that visit.
The location of the temporary file is determined by a setting in the php.ini file: session.save_path. Its current value is shown in the session block of the phpinfo.php page.
Let's check what can happen when a session is started:
- PHP creates a unique identifier for the session. The session ID or session token is a string of 32 hexadecimal numbers.
- A cookie called PHPSESSID is automatically sent to the user's computer to store unique session identification.
- A file is automatically created on the server and has the name of the unique identifier prefixed by sess_.
Now data can be stored in session variables since the session is established. The data will be recorded in the sess_ file on the server.
When a script tries to retrieve the value from a session variable, PHP automatically gets the unique session ID string from the PHPSESSID cookie. Then it looks into temporary directory for the file that has the same name. Once this is found, the file is opened and the variable is recovered.
As this process relies upon only the cookie on the user's computer and the file in the server's temporary directory, the session variables are available to each page of the entire site. The session ends when the user closes their browser or does not load a new page for a predetermined period of time.
Here is the Firefox Cookie example:
Tools > Options..., then we get
Select Use custom settings..., then we'll have a new items on the Options dialog.
If we click Show Cookies... button, we get the infomation about the previous visits.
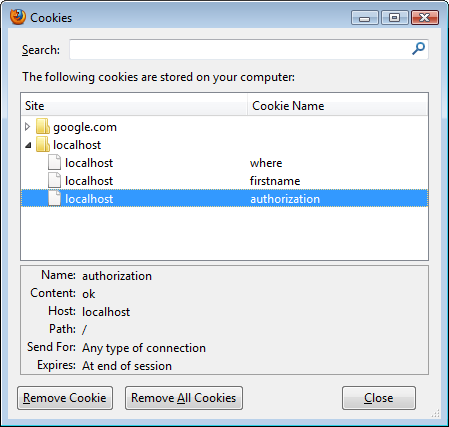
A PHP session is started by making a call to the session_start() function. It checks to see if a session has already been started, and then starts one if nothing exists. It also tells the PHP engine to expect session variables to be used in the scripts on this page. Thus, the call to that function should be at the beginning of the page.
A session variable is referenced by the $_SESSION[] array variable. For example, $_SESSION['visit_count'] = 1 initializes a session variable named "visit_count" with a value of 1.
The example below uses the isset() function to first establish if a particular session variable already exists. If it's not there, the script creates the session variable named visit_count. Each time the page is visited during the session, the visit_count values gets incremented by 1.
#visit_count.php <?php session_start(); if( isset( $_SESSION['visit_count']) ) $_SESSION['visit_count']++; else $_SESSION['visit_count'] = 1; ?> <html> <head> <title>Counting Visit</title> </head> <body> <p>You have visited this page <?php echo $_SESSION['visit_count']; ?> time(s) in this session.</p> </body> </html>
Before we run the script, let's look at the cookie setting for IE.
Tools > Internet Options > Privacy Tab.
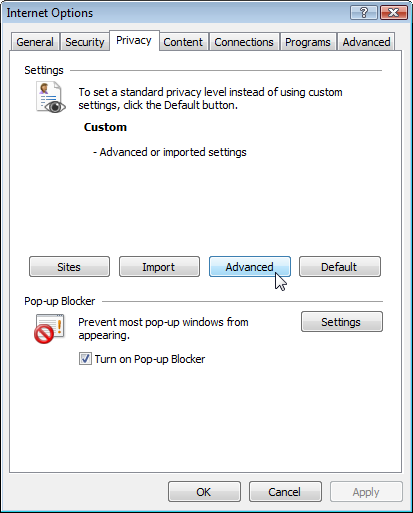
Click Advance button, and block all cookies as in the picture below.
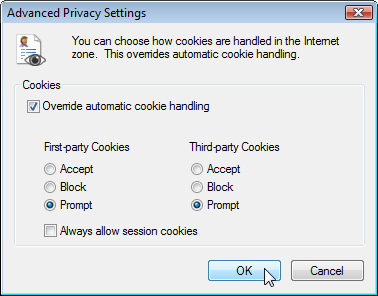
Now let's try to run the script, open IE and type localhost/visit_count.php into the URL. Then, we'll get:
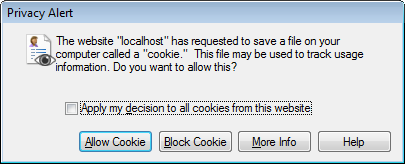
Before we allow click, let's click the More Info button.
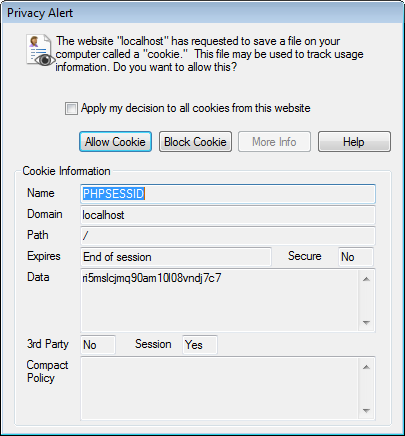
Now, let's allow cookie by pressing Allow Cookie button. Then we get an output:
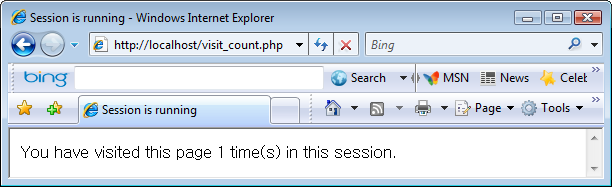
Run it again by refreshing it. We get a message saying we've been visited 2nd time.
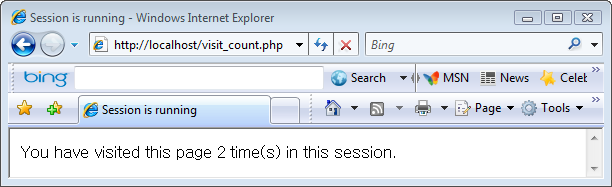
When the page loads, the value of the variable visit_count incremented by one. The name and value of this variable are stored in the associated file in the temporary directory on the server. Each time the page is reloaded the value is retrieved from the file, and then incremented and the updated value is stored.
The example demonstrated how the PHPSESSID cookie is used to store the unique ID required by the session process. This may seem to be redundant: store and retrieve data.
However, there is another way to make the ID accessible across an entire website without requiring the browser to accept cookies. This method appends the ID to the URL in each hyperlink to other pages on that website.
The new method is explained in next chapter.
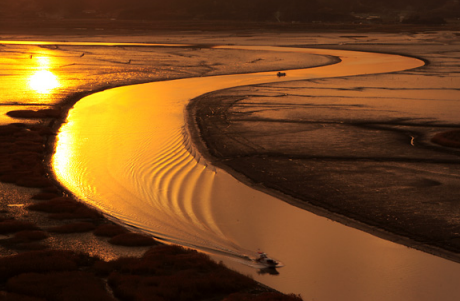
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization