PHP & MySQL Tutorial - Request 2015
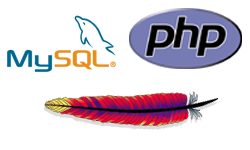
PHP is designed to generate web pages interactively on a web server.
Unlike HTML, where the web browser uses tags and markup to generate a page, PHP code runs between the requested page and the web server.
While PHP is great for web application development, it doesn't store information by itself. Because of that, we need a database, MySQL. PHP and MySql have been developed with each other in mind. The programming interfaces between them are logically paired up.
The current release of MySQL is the 5.x version. MySQL 5.6 provides performance that is comparable to other databases such as Oracle, Informix, DB2 (IBM), and SQL Server (Microsoft).
Apache is a web server and has two major versions in use today: 1.3 and 2. Apache 2 is a major rewrite and supports threading though PHP isn't totally ready yet.
Whenever I try to learn a new language, I usually want to know what I can do with it in the end before I start learning the language syntax in detail. So, I am going to try the same thing here.
I'll make a sample application for a new user signing up on a site.
The signup form looks like this:
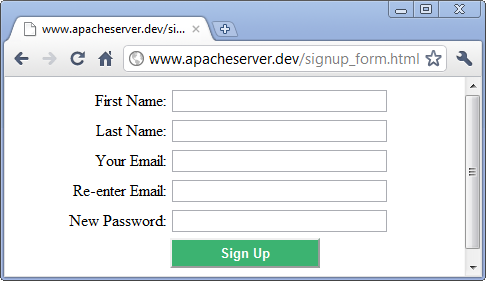
The htlm code for the form is signup_form.html:
<html> <body> <form action="process_signup.php" method="post"> <table border="0"> <tr> <td width="150" align="right">First Name:</td> <td align="center"> <input type="text" name="firstname" size="30" maxlength="30"/></td> </tr> <tr> <td width="150" align="right">Last Name:</td> <td align="center"> <input type="text" name="lastname" size="30" maxlength="30"/></td> </tr> <tr> <td width="150" align="right">Your Email:</td> <td align="center"> <input type="text" name="email" size="30" maxlength="30"/></td> </tr> <tr> <td width="150" align="right">Re-enter Email:</td> <td align="center"> <input type="text" name="reenteremail" size="30" maxlength="30"/></td> </tr> <tr> <td width="150" align="right">New Password:</td> <td align="center"> <input type="text" name="newpassword" size="30" maxlength="30"/></td> </tr> <tr> <td/> <td align="left"><input type="submit" value="Sign Up" style="background:mediumseagreen; color:#ffffff; font-weight:bold; width:150px; height:30px"/></td> </tr> </table> </form> </body> </html>
After processing the signup, we get the following page.
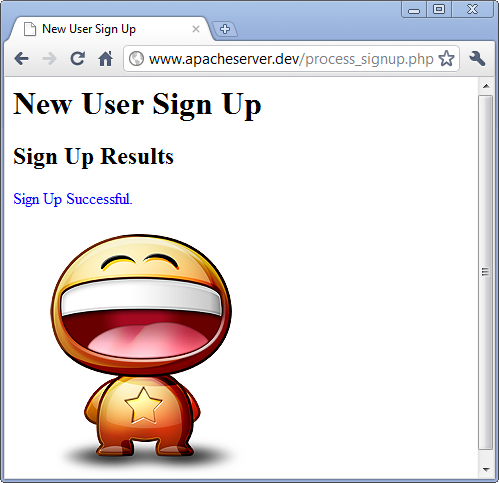
To process the form, we need to create the php script (process_signup.php) mentioned in the action attribute of the form tag.
It looks like this:
<html> <head> <title>New User Sign Up</title> </head> <body> <h1>New User Sign Up</h1> <h2>Sign Up Results</h2> <?php echo '<p><font color="blue">Sign Up Successful.</font></p>'; echo '<img src="images/php1/happy_face.png" alt="happy face"/>'; ?> </body> </html>
Actually, we did not process any user sign-up and it requires more PHP code in between "<?php" and "?>".
What we've done is demonstrating the embedded PHP inside HTML processing the HTML code inside echo.
So, how the PHP code was embedded inside HTML file?
Let's look at the code using view source feature of the browser:
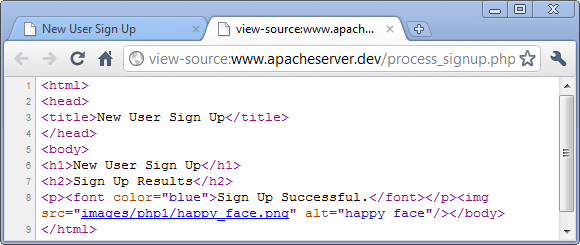
Notice that none of the raw PHP is visible because the PHP interpreter has run through the script and replaced it with the output from the script. This means that from PHP we can produce HTML code which is viewable with any browser. So, the user's browser does not need to understand PHP.
We've just seen so-called server-side scripting. The PHP has been interpreted and executed on the web server when the user requests a document. PHP produces output in a format understandable by web browsers (usually HTML), which is then sent to the user's computer. The user cannot see the script's source code (as we saw from the picture above), and may not even be aware that a script was executed. Documents produced by server-side scripts may, in turn, contain client-side scripts.
Compared with the client-side scripts which have greater access to the information and functions available on the user's browser, server-side scripts have greater access to the information and functions available on the server.
Here is a simple diagram shows the process of the server-side scripting.
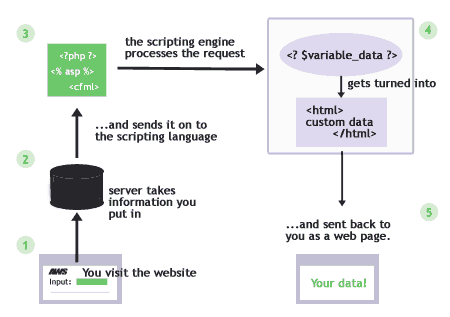
We should always use <?php and ?> to delimit our PHP code. This is the most portable and supported format. Don't use the older <? and ?> tags, as they're not fully supported and can confuse XML parsers.
Basically, the tags tell the web server where the PHP code starts and finishes. Any text between the tags is considered as PHP and any text outside the tags is interpreted as normal HTML. In essence, the PHP tags allow us to escape from HTML.
Dynamic web page means that the user interacts with the web site beyond just reading pages, and the web site responds accordingly. The main reason for using a server-side scripting language is to be able to provide dynamic content to the users.
Not long ago, creating dynamic web pages meant writing a lot of code in the C or Perl, and then calling and executing those programs through a Common Gateway Interface (CGI). But PHP and MySQL make creating dynamic web sites easier and faster.
In order to process and develop dynamic web pages, we'll need to use and understand several technologies. There are three main components of creating dynamic web pages:
- A web server
- A server-side programming language
- A database
Following two pictures show the relationship of those three components of dynamic web page listed above.
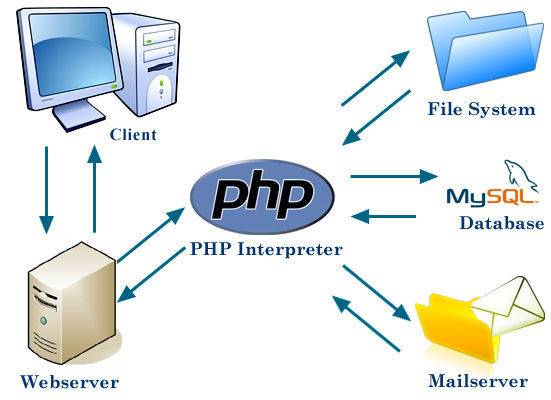
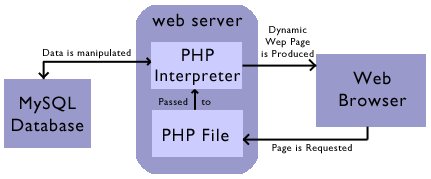
Let's make a simple example. Modify the process_signup.php like this:
<html> <head> <title>New User Sign Up</title> </head> <body> <h1>New User Sign Up</h1> <h2>Sign Up Results</h2> <?php date_default_timezone_set('America/Los_Angeles'); echo date('H:i, jS F Y'); echo '<p><font color="red">Sign Up Successful!</font></p>'; echo '<img src="images/php1/happy_face.png" alt="happy face"/>'; ?> </body> </html>
The resulting HTML page is almost the same as before except we now have time stamp on it.
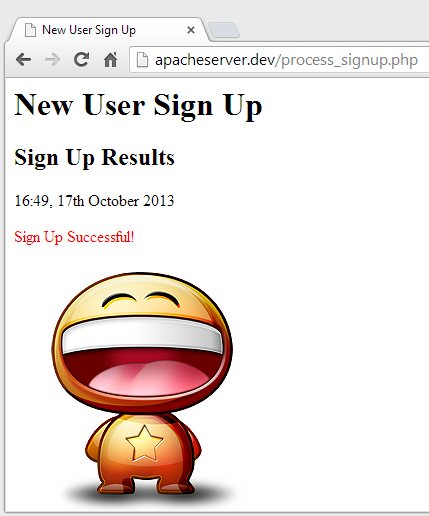
The PHP's date() function tells the customer the data and time when his signup was successfully processed. This information will be different each time the PHP script is run.
Here is the description of the date() function:
string date ( string $format [, int $timestamp ] )
It returns a string formatted according to the given format string using the given integer timestamp or the current time if no timestamp is given. In other words, timestamp is optional and defaults to the value of time().
Note that before the call data(), we set the time zone using date_default_timezone_set() function:
date_default_timezone_set('America/Los_Angeles');
In the next section, we'll process the information that the user typed in.
And we'll learn how to access form variables.
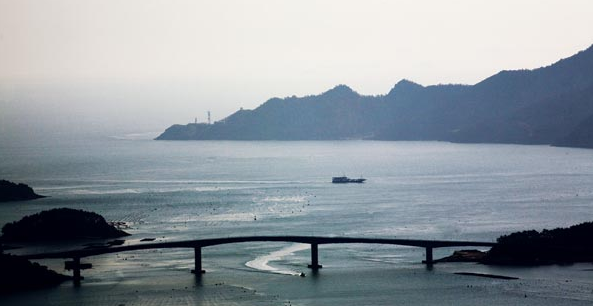
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization