PHP & MySQL Tutorial
- Arrays II
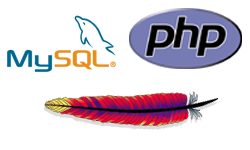
Additional elements can be created at the beginning of an array using the array_unshift() function, and elements can be added at the end of an array with the array_push() function.
<?php $numbers = array(2,3,4); foreach($numbers as $n) { echo "$n" . " "; } echo "\n"; array_unshift($numbers,0,1); foreach($numbers as $n) { echo "$n" . " "; } echo "\n"; array_push($numbers,5); foreach($numbers as $n) { echo "$n" . " "; } ?>
Output is:
2 3 4 0 1 2 3 4 0 1 2 3 4 5
Each requires the array name followed by the element data as its arguments.
In the example below, an array is created with five elements. Two more elements are added at the beginning of the array, then three more elements are added at the end.
<html> <head><title>Adding Arrays</title></head> <body> <?php $composers = array("Ludwig van Beethoven", "Piotr Il'yich Tchaikovsky", "Igor Stravinsky", "Richard Wagner", "Johann Sebastian Bach"); # add elements at the beginning array_unshift($composers, "Claude Debussy", "Arnold Schoenberg"); # add elements at the end array_push($composers, "Modest Mussorgsky", "Wolfgang Amadeus Mozart", "Gabriel Fauré"); echo "<p><b>The Greatest Composers</b></p><ul>"; foreach ($composers as $value) { echo "<li>$value</li>"; } echo "</ul>"; ?> </body> </html>
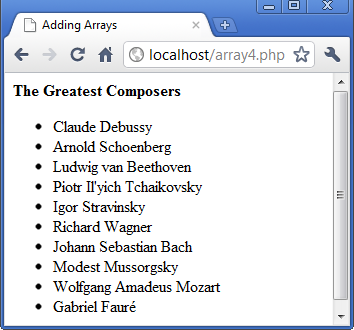
The first element in an array can be removed with the array_shift() function, and the final element can be removed using array_pop() function. Both of these functions return the removed element data, which can be assigned to a variable.
<?php $numbers = array(1,2,3,4,5); foreach($numbers as $n) { echo "$n" . " "; } echo "\n"; array_shift($numbers); foreach($numbers as $n) { echo "$n" . " "; } echo "\n"; array_shift($numbers); foreach($numbers as $n) { echo "$n" . " "; } echo "\n"; array_pop($numbers); foreach($numbers as $n) { echo "$n" . " "; } ?>
The output is:
1 2 3 4 5 2 3 4 5 3 4 5 3 4
The example below removes the first and last elements from an array, then using sort() function, the array will be sorted in alphabetical order.
<html> <head><title>Removing and Sorting Arrays</title></head> <body> <?php $composers = array("Ludwig van Beethoven", "Piotr Il'yich Tchaikovsky", "Igor Stravinsky", "Richard Wagner", "Johann Sebastian Bach"); # add elements at the beginning array_unshift($composers, "Claude Debussy", "Arnold Schoenberg"); # add elements at the end array_push($composers, "Modest Mussorgsky", "Wolfgang Amadeus Mozart", "Gabriel Faure"); echo "<p><b>The Greatest Composers</b></p><ul>"; foreach ($composers as $value) { echo "<li>$value</li>"; } echo "</ul>"; # remove 1st element $first = array_shift($composers); echo "<p>$first is removed <br />"; # remove last element $last = array_pop($composers); echo "$last is removed </p>"; # sort sort($composers); # array size $size = count($composers); echo "<p><b>After removing and sorting $size composers</b></p><ul>"; foreach ($composers as $value) { echo "<li>$value</li>"; } echo "</ul>"; ?> </body> </html>
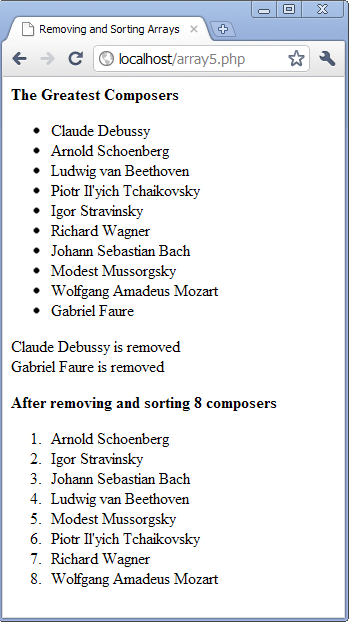
Arrays in PHP can be manipulated by the special array functions.
The array_merge() function makes two arrays to be merged with the two array names as its arguments. This will add the elements of the second array after those of the first array.
A specified range of an array's elements can be selected using the array_slice function. It takes the array name and the start and end locations of the required elements.
The shuffle() function can randomly rearrange an array elements.
Following example shows how we can use the functions mentioned above.
<html> <head><title>Special Array Functions</title></head> <body> <?php $popSongsA = array( "John Lennon - \"Imagine\" (1971)", "Judy Garland - \"Over the Rainbow\" (1939)", "Beatles - \"Yesterday\" (1965)", "Righteous Brothers - \"Unchained Melody\" (1965)", "Joan Jett and the Blackhearts - \"I Love Rock 'n Roll\" (1982)"); $popSongsB = array( "Kelly Clarkson - \"Since U Been Gone\" (2004)", "Simon & Garfunkel - \"Mrs. Robinson\" (1968)", "A-Ha - \"Take On Me\" (1984)", "Michael Jackson - \"Billie Jean\" (1983)", "Stevie Wonder - \"Superstition\" (1972)"); $mySongList = array_merge($popSongsA, $popSongsB); echo "<h1>My Favorite Songs</h1>"; echo "<ul>"; foreach ($mySongList as $value) { echo "<li>$value</li>"; } echo "</ul>"; $mySongList2 = array_slice($mySongList,4,3); echo "<br /><h3>Sliced List</h3>"; echo "<ul>"; foreach ($mySongList2 as $value) { echo "<li>$value</li>"; } echo "</ul>"; srand((float)microtime()*1000000); shuffle($mySongList); echo "<br /><h3>Shuffled List</h3>"; echo "<ul>"; foreach ($mySongList as $value) { echo "<li>$value</li>"; } echo "</ul>"; ?> </body> </html>
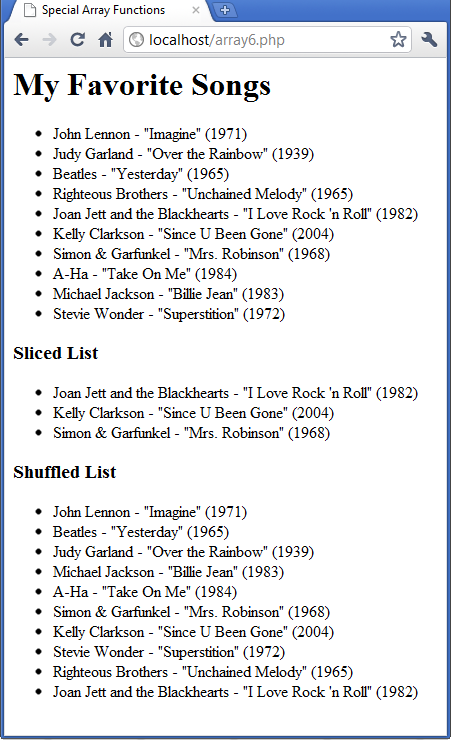
Following are the most common functions:
- Reset(array)
Takes an array as its argument and resets the pointer to the beginning of the array. The pointer is how PHP keeps track of the current element in an array when working with functions that can move around in arrays. The value of the first element is also returned. - Array_push(array, elements)
Adds one or more elements to the end of an existing array. For instance,array_push($composers, "Ludwig van Beethoven", "Piotr Il'yich Tchaikovsky", "Igor Stravinsky");
adds those three elements to an array called $composers. - Array_pop(array)
Returns and removes the last element of an array.$last_element = array_pop($composers);
removes the last element from $composers and assigns it to @last_element. - Array_unshift(array, elemens)
Adds one or more elements to the beginning of an existing array. For example,array_unshift($composers,"Ludwig van Beethoven", "Piotr Il'yich Tchaikovsky", "Igor Stravinsky");
adds three elements to the beginning of an array called $composers. - Array_shift(array)
Returns and removes the first element of an array. For example,$first_element = array_shift($composers);
removes the first element from an array called $composers and assigns it to $first_element. - Array_merge(array,array)
Combines two arrays together and returns the new array. For example,$combined_array = array_merge($composers,$writers);
combines the elements of both arrays and assigns the new array to $combined_array. - Array_keys(array)
Returns an array containing all of the keys from the supplied array. - Array_values(array)
Returns a numerically indexed array containing all of the values from the supplied array. - Shuffle(array)
Resorts the array in random order. The key values are lost when the array is shuffled because the returned array is a numeric array.
We can convert between arrays and strings using implode() and explode() functions.
The implode() function converts an array to a string and inserts a specified separator between each value. Usually, this can be used to generate a list for cvs.
explode() can be used to form an array from a string. It returns an array of strings created by splitting the string by a delimiter.
The other one is list. It returns an array of strings created by splitting the string parameter by a delimiter.
<?php $numbers = array(1,2,3,4,5); foreach($numbers as $n) { echo "$n" . ","; } echo "\n"; // instead of using foreach(), we can use implode() $csv = implode(',', $numbers); echo $csv; echo "\n"; // convert it back to array $numbers2 = explode(',', $csv); foreach($numbers2 as $n2) { echo "$n2" . " "; } echo "\n"; $str = "1:2:3:4:5"; // list returns an array of strings list($one, $two, $three, $four, $five) = explode(':', $str); echo $one . $two . $three . $four . $five; ?>
Output is:
1,2,3,4,5, 1,2,3,4,5 1 2 3 4 5 12345
<html> <head><title>List and Explode</title></head> <body> <?php $composers = "Ludwig van Beethoven/Piotr Il'yich Tchaikovsky/ Igor Stravinsky/Richard Wagner/Johann Sebastian Bach"; $each_composer = explode("/", $composers); echo "<ul>"; foreach ($each_composer as $value) { echo "<li>$value</li>"; } echo "</ul>"; $address = "192.1.21.38"; list($ip1,$ip2,$ip3,$ip4) = explode(".", $address); echo "<ul>"; echo "<li>$ip1</li>"; echo "<li>$ip2</li>"; echo "<li>$ip3</li>"; echo "<li>$ip4</li>"; echo "</ul>"; ?> </body> </html>
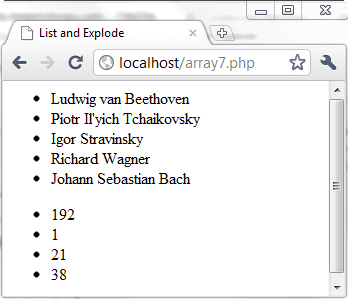
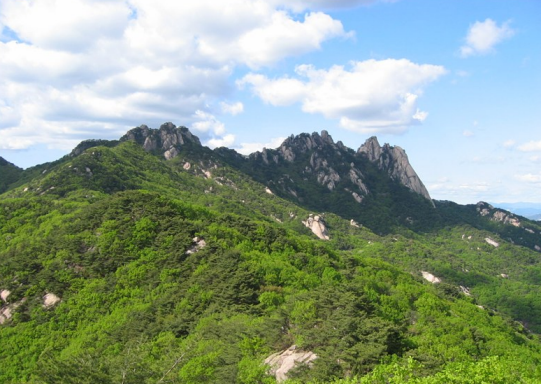
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization