PHP & MySQL Tutorial
Creating Dynamic Content III
- PHP_SELF
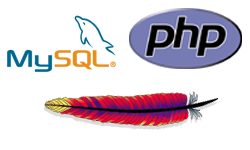
In this chapter, we'll see how to use PHP_SELF variable.
The examples so far have used a different file as a form handler to process submitted data, but the same page can be used as the form handler by assigning the PHP environment variable called $_SERVER['PHP_SELF'] to the form's action attribute.
PHP_SELF is a variable that returns the current script being executed. This variable returns the name and path of the current file (from the root folder). You can use this variable in the action field of the form.
What's the result of this?
echo $_SERVER['PHP_SELF'];
It displays the name and path of the current file (from the root folder). The following file is phpself.php
<html> <head><title>PHP_SELF</title></head> <body> <?php echo $_SERVER['PHP_SELF']; ?> </body> </html>
If we put it in the root directory (C:/server/www/apacheserver.dev/public_html), the output will be:
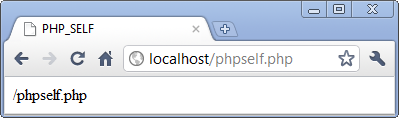
If it's in C:/server/www/apacheserver.dev/public_html/subdir/, the output we get:
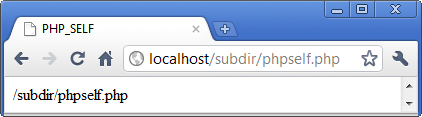
A common use of PHP_SELF variable is in the action field of the <form> tag.
The action field of the FORM instructs where to submit the form data when the user presses the submit button. It is common to have the same PHP page as the handler for the form as well.
However, if you provide the name of the file in the action field, in case you happened to rename the file, you need to update the action field as well. Or else your forms will stop working.
Consider, you have a file called form-action.php and want to load the same page after the form is submitted. The usual form code will be:
<form method="post" action="form-action.php" >
We can use the PHP_SELF variable instead of form-action.php. The code becomes:
<form method="post" action="<?php echo $_SERVER['PHP_SELF']; ?>" >
Here is the combined code, reload_form.php that contains both the form and the PHP script.
<?php if(isset($_POST['submit_button'])) { $nameTypedIn = $_POST['userName']; echo "User has submitted the form and entered this name : <b> $nameTypedIn </b>"; echo "<br>You can reload this form to enter a new name."; } ?> <html> <head><title>Using PHP_SELF</title></head> <body> <form method="post" action="<?php echo $_SERVER['PHP_SELF']; ?>"> Name: <input type="text" name="userName"><br> <input type="submit" name="submit_button" value="Submit Form"><br> </form> </body> </html>
This PHP code is above the HTML part and will be executed first. The first line of code is checking if the form is submitted or not. The name of the submit button is submit_button. When the submit button is pressed the $_POST['submit_button'] will be set and the if condition will become true. In this case, we are showing the name entered by the user. If the form is not submitted the if condition will be false as there will be no values.
When it's loaded first time, we have the form looks like this:
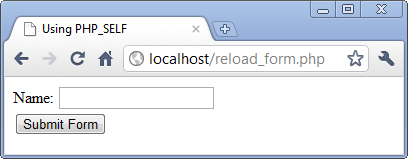
Then, we typed in a name Donald E. Knuth:
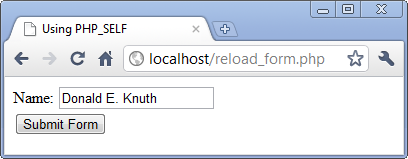
After we submit, the form we get should look like this:
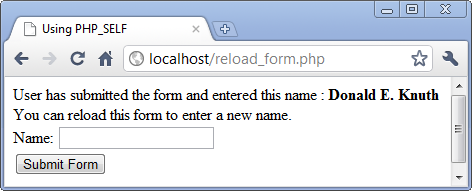
We can type a new name and re-submit, then the form we get is:
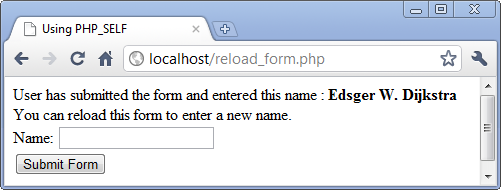
The PHP header() function provides raw HTTP headers to the browser and can be used to redirect it to another location. The redirection script must be at the very top of the page to avoid any other part of the page from loading.
The target is specified by using the Location: header which is the argument to the header() function. After calling this function, the exit() function may be used to halt parsing of the code on that page.
In the following example, a drop-down selection box provides the user a several locations to visit. When the form is submitted, the page is reloaded and the $location variable is used to redirect the browser.
# redirectingSites.php <?php if(isset($_POST['submit_button'])) { $site_to_visit = $_POST['site']; $self = $_SERVER['PHP_SELF']; if( $site_to_visit != null ) { header( "Location:$site_to_visit") ; exit(); } } ?> <html> <head><title>Redirect</title></head> <body> Choose a site to visit: <form action = "<?php $self ?>" method = "post"> <select name = "site"> <option value = "http://www.bogotobogo.com">Web Animation</option> <option value = "http://en.wikipedia.org/wiki/PHP">PHP - Wikipedia, the free encyclopedia</option> <option value = "http://www.apache.org">The Apache Software Foundation</option> <option value = "http://www.mysql.com">MySQL</option> <option value = "http://www.linux.org">The Linux Home Page</option> <option value = "http://www.pbase.com/dbh/soraksan">Mt.Sorak National Park </option> <input type = "submit" name = "submit_button" value = "Go"> </form> </body> </html>
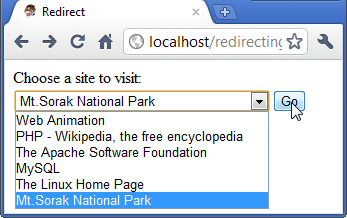
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization