PHP Tutorial Laravel 4 Framework (Authentication) - Changing Password I
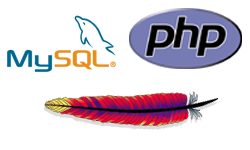
Laravel 4
- Installing on Ubuntu - local
- Installing on a Shared host
- Installing on Windows
- Creating users table
- Home page with controller and blade
- Blade Templating
- Database connection and sending emails
- Creating user account I - GET
- Creating user account II - POST
- Creating user account III - Error checking & redirecting input
- Creating user account IV - User::create()
- Activating user account I - Mail::send()
- Activating user account II - Update user's status
- User account sign-in I - Route and link
- User account sign-in II - Validation
- User account sign-in III - Login Authentication
- Singing out
- Remember the user
- Changing Password I
- Changing Password II
- Recovering forgotten password I
- Recovering forgotten password II
- User Profile
- Database Migration using artisan
This tutorial is the continuation from Remember.
In this chapter, we'll write a code so that users can change their passwords.
In this section, we'll provide a check box so that a user can have a choice for the remember feature.
views/layout/navigator.blade.php file:
<nav> <ul> <li> <a href="{{ URL::route('home') }}">Home</a></li> @if(Auth::check()) <li><a href="{{ URL::route('account-sign-out') }}">Sign out</a></li> <li><a href="">Change password</a></li> @else <li><a href="{{ URL::route('account-sign-in') }}">Sign in</a></li> <li><a href="{{ URL::route('account-create') }}">Create an account</a></li> @endif </ul> </nav>
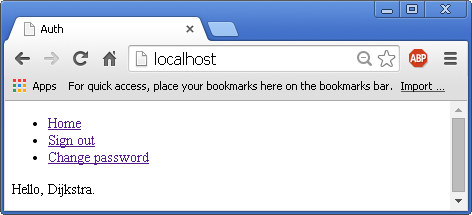
Now we have a link for "Change password". But it's just a link not going anywhere. So, we need more work.
We will provide route with the link for Change password in routes.php file:
/* Authenticated group */ Route::group(array('before' => 'auth'), function() { // Change password (GET) Route::get('/account/change-password', array('as' => 'account-change-password', 'uses' => 'AccountController@getChangePassword' )); ... });
We need to provide the view (forms) in a new file password.blade.php;
@extends('layout.main') @section('content') Change password. @stop
Url to our controller:
<nav> <ul> <li> <a href="{{ URL::route('home') }}">Home</a></li> @if(Auth::check()) <li><a href="{{ URL::route('account-sign-out') }}">Sign out</a></li> <li><a href="{{ URL::route('account-change-password') }}">Change password</a></li> @else <li><a href="{{ URL::route('account-sign-in') }}">Sign in</a></li> <li><a href="{{ URL::route('account-create') }}">Create an account</a></li> @endif </ul> </nav>
We haven't written the get method for password change:
public function getChangePassword() { return View::make('account.password'); }
When we click the link, "Change password", we should get the simple text output of "Change password" on http://localhost/account/change-password page.
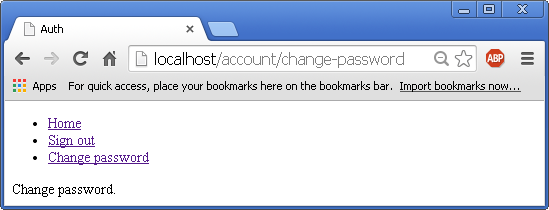
Before we make the change password forms, we need to provide the route for post submit route in routes.php:
/* Authenticated group */ Route::group(array('before' => 'auth'), function() { /* CSRF protection */ Route::group(array('before' => 'csrf'), function() { /* Change password (POST) */ Route::post('/account/change-password', array('as' => 'account-change-password-post', 'uses' => 'AccountController@postChangePassword' )); }); // Change password (GET) Route::get('/account/change-password', array('as' => 'account-change-password', 'uses' => 'AccountController@getChangePassword' ));
Now, we have both GET and POST routes. Let's build the forms next.
In views/account/password.blade.php:
@extends('layout.main') @section('content') <form action="{{ URL::route('account-change-password-post') }}" method="post"> <input type="submit" value="Change password"> {{ Form::token() }} </form> @stop
We need POST method in our AccountController.php:
public function postChangePassword() { }
Let's check what we've got so far:
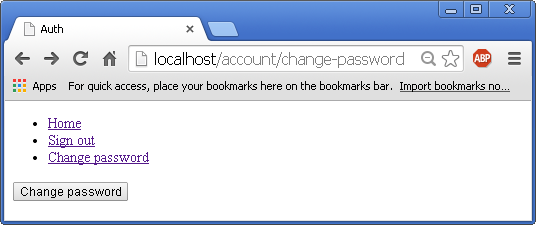
If we submit, we get blank page which is expected. But important thing here is we get no errors.
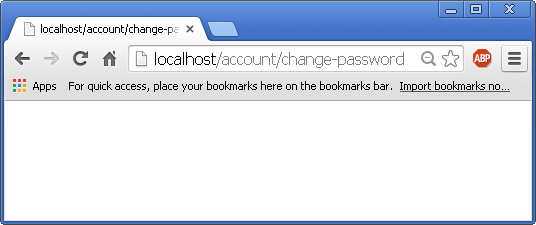
Laravel 4
- Installing on Ubuntu - local
- Installing on a Shared host
- Installing on Windows
- Creating users table
- Home page with controller and blade
- Blade Templating
- Database connection and sending emails
- Creating user account I - GET
- Creating user account II - POST
- Creating user account III - Error checking & redirecting input
- Creating user account IV - User::create()
- Activating user account I - Mail::send()
- Activating user account II - Update user's status
- User account sign-in I - Route and link
- User account sign-in II - Validation
- User account sign-in III - Login Authentication
- Singing out
- Remember the user
- Changing Password I
- Changing Password II
- Recovering forgotten password I
- Recovering forgotten password II
- User Profile
- Database Migration using artisan
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization