Bottle micro web services framework 2 : static files
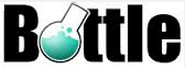
List of Bottle Micro Web Services Tutorials
- Introduction
- Static files
- Template
- json
- Bucket List App I - sqlite, route, and template
- Bucket List App II - get & post
- Bucket List App III - Editing
- Bucket List App IV - route validation, regex, and static_file
- Bucket List App V - json
- json to html table
- Forms - Get & Post
- Forms - Get & Post with editable and checkbox table cells
static_files.py:
from bottle import route, run, static_file @route('/') def serve_homepage(): return static_file('home.html', root='static/') run(host='localhost', port=8080)
Run the script file:
$ python static_files.py Bottle v0.12.7 server starting up (using WSGIRefServer())... Listening on http://localhost:8080/ Hit Ctrl-C to quit. 127.0.0.1 - - [04/Oct/2014 11:44:24] "GET / HTTP/1.1" 200 39
Here is the rendered page:
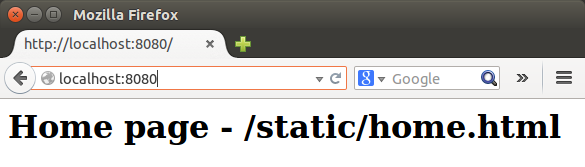
st_file.py:
from bottle import route, run, static_file @route('/static/<filename:path>') def st(filename): return static_file(filename, root='static/') run(host='localhost', port=8080)
static/myfile:
This is a file in /static/myfile.
Run the script file:
$ python st_file.py Bottle v0.12.7 server starting up (using WSGIRefServer())... Listening on http://localhost:8080/ Hit Ctrl-C to quit. 127.0.0.1 - - [04/Oct/2014 15:48:30] "GET /static/myfile HTTP/1.1" 304 0
Here is the rendered page:

Let's start with a simple html page:
main.html:
<!DOCTYPE html> <html lang="en"> <head> <title>My Bottle Home</title> <link rel="stylesheet" type="text/css" href="http://twitter.github.com/bootstrap/assets/css/bootstrap.css"> </head> <body> <h1>Initial Home page</h1> <p>My image:</p> <img src="images/bottle.png"> </body> </html>
main.py:
from bottle import route, run, static_file, template HOST = 'localhost' @route('/') def serve_homepage(): return template('main.html') run(host=HOST, port=8080, debug=True)
Run the script file:
$ python main.py Bottle v0.12.7 server starting up (using WSGIRefServer())... Listening on http://localhost:8080/ Hit Ctrl-C to quit. 127.0.0.1 - - [04/Oct/2014 20:42:55] "GET / HTTP/1.1" 200 248-
Here is the rendered page:
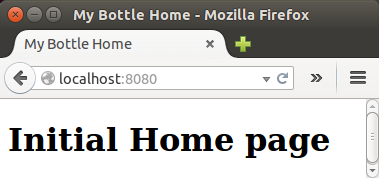
We get the same result if we rename the main.html to main.tpl, and return the tpl instead of html.
main.py:
from bottle import route, run, static_file, template HOST = 'localhost' @route('/') def serve_homepage(): return template('main.tpl') run(host=HOST, port=8080, debug=True)
However, note that we're missing an image and the rendered page should look like this:
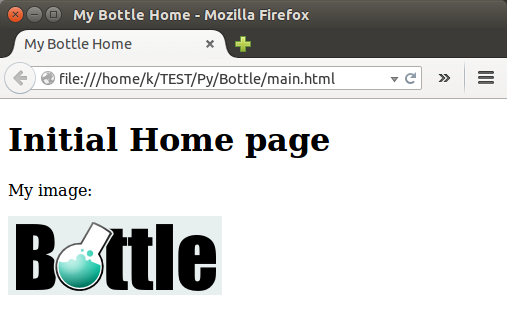
Let's make it work. Here is main.py we're using:
from bottle import route, run, static_file, template HOST = 'localhost' @route('/static/<filepath:path>') def server_static(filepath): return static_file(filepath, root='/home/k/TEST/Py/Bottle/') @route('/') def serve_homepage(): return template('main.tpl') run(host=HOST, port=8080, debug=True)
Note that the path ('/home/k/TEST/Py/Bottle/') in
return static_file(filepath, root='/home/k/TEST/Py/Bottle/')
is the site root directory.
main.tpl:
<!DOCTYPE html> <html lang="en"> <head> <title>My Bottle Home</title> <link rel="stylesheet" type="text/css" href="http://twitter.github.com/bootstrap/assets/css/bootstrap.css"> </head> <body> <h1>Initial Home page</h1> <p>My image:</p> <img src="/static/bottle.png"> </body> </html>
Here we append the '/static/' before the 'bottle.png' image.
Then, run the server:
k@k:~/TEST/Py/Bottle$ python main.py Bottle v0.12.7 server starting up (using WSGIRefServer())... Listening on http://localhost:8080/ Hit Ctrl-C to quit. 127.0.0.1 - - [05/Oct/2014 13:27:41] "GET / HTTP/1.1" 200 304 127.0.0.1 - - [05/Oct/2014 13:27:41] "GET /static/bottle.png HTTP/1.1" 304 0
Now, the new page will have the image rendered properly:
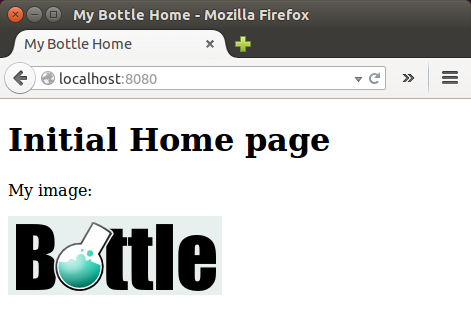
If we move the 'bottle.png' file from the 'root' directory into a subdirectory such as images, and modify main.tpl like this:
<p>My image in '/home/k/TEST/Py/Bottle/images':</p> <img src="/static/images/bottle.png">
we still get the image properly rendered page:
$ python main.py Bottle v0.12.7 server starting up (using WSGIRefServer())... Listening on http://localhost:8080/ Hit Ctrl-C to quit. 127.0.0.1 - - [05/Oct/2014 14:50:51] "GET / HTTP/1.1" 200 311 127.0.0.1 - - [05/Oct/2014 14:50:51] "GET /static/images/bottle.png HTTP/1.1" 200 7300 127.0.0.1 - - [05/Oct/2014 14:50:52] "GET /favicon.ico HTTP/1.1" 404 742
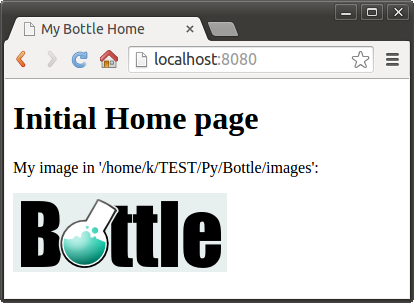
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization