Flask blog app tutorial 3 : Adding blog item
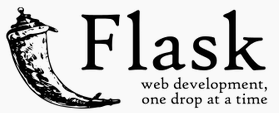
In the previous part of this series, we implemented the sign-in and sign-out.
In this part of the series, we'll let user add a blog post.
Here are the files we'll be using in this tutorial part-3:
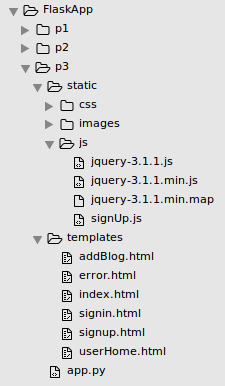
They are available from FlaskApp/p3
We'll start by creating an interface for the logged-in user to add a blog post. Let's create a file addBlog.html:
<!DOCTYPE html> <html lang="en"> <head> <title>Python Flask Blog App</title> <link href="//getbootstrap.com/dist/css/bootstrap.min.css" rel="stylesheet"> <link href="//getbootstrap.com/examples/jumbotron-narrow/jumbotron-narrow.css" rel="stylesheet"> <script src="/static/js/jquery-3.1.1.js"></script> </head> <body> <div class="container"> <div class="header"> <nav> <ul class="nav nav-pills pull-right"> <li role="presentation" class="active"><a href="#">Add Item</a></li> <li role="presentation" ><a href="/logout">Logout</a></li> </ul> </nav> <img src="/static/images/Flask_Icon.png" alt="Flask_Icon.png"/ > </div> <section> <form class="form-horizontal" method="post" action="/addBlog"> <fieldset> <!-- Form Name --> <legend>Create Your Blog</legend> <!-- Text input--> <div class="form-group"> <label class="col-md-4 control-label" for="txtTitle">Title</label> <div class="col-md-4"> <input id="txtTitle" name="inputTitle" type="text" placeholder="placeholder" class="form-control input-md"> </div> </div> <!-- Textarea --> <div class="form-group"> <label class="col-md-4 control-label" for="txtPost">Post</label> <div class="col-md-4"> <textarea class="form-control" id="txtPost" name="inputDescription" ></textarea> </div> </div> <!-- Button --> <div class="form-group"> <label class="col-md-4 control-label" for="singlebutton"></label> <div class="col-md-4"> <input id="singlebutton" name="singlebutton" class="btn btn-primary" type="submit" value="Publish" /> </div> </div> </fieldset> </form> </section> <footer class="footer"> <p>©etaman.com 2017</p> </footer> </div> </body> </html>
Now, we may want to add a new route and method to display the "Add Blog" page in app.py:
@app.route('/showAddBlog') def showAddBlog(): return render_template('addBlog.html')
We need to add a new menu item to link to the "Add Blog" page in userHome.html:
<nav> <ul class="nav nav-pills pull-right"> <li role="presentation"><a href="/showAddBlog">Add Blog</a></li> <li role="presentation" class="active"><a href="/logout">Logout</a></li> </ul> </nav>
When the user logged in successfully, the following page is presented:
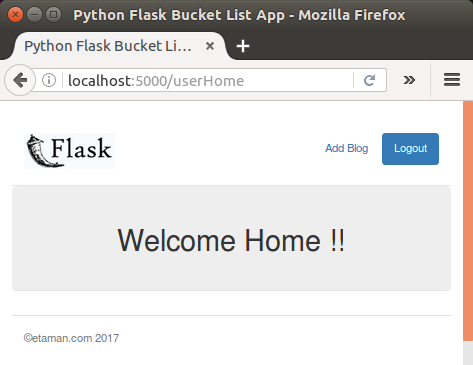
When the user click "Add Blog", the page below will show up:
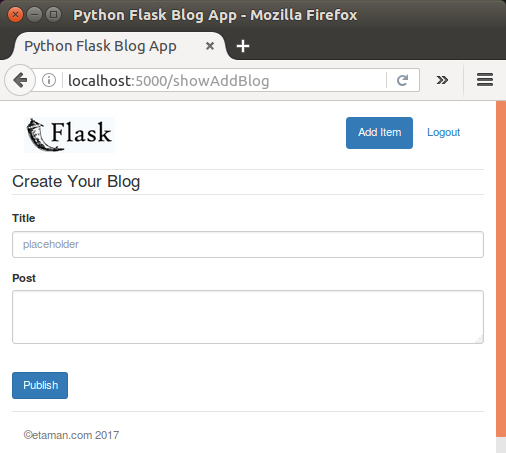
In order to add items to the blog list, we need to create a table called tbl_blog:
CREATE TABLE `tbl_blog` ( `blog_id` int(11) NOT NULL AUTO_INCREMENT, `blog_title` varchar(45) DEFAULT NULL, `blog_description` varchar(5000) DEFAULT NULL, `blog_user_id` int(11) DEFAULT NULL, `blog_date` datetime DEFAULT NULL, PRIMARY KEY (`blog_id`) ) ENGINE=InnoDB AUTO_INCREMENT=3 DEFAULT CHARSET=latin1;
Check our tables so far:
mysql> show tables; +------------------------+ | Tables_in_FlaskBlogApp | +------------------------+ | blog_user | | tbl_blog | +------------------------+ 2 rows in set (0.00 sec) mysql> describe tbl_blog; +------------------+---------------+------+-----+---------+----------------+ | Field | Type | Null | Key | Default | Extra | +------------------+---------------+------+-----+---------+----------------+ | blog_id | int(11) | NO | PRI | NULL | auto_increment | | blog_title | varchar(45) | YES | | NULL | | | blog_description | varchar(5000) | YES | | NULL | | | blog_user_id | int(11) | YES | | NULL | | | blog_date | datetime | YES | | NULL | | +------------------+---------------+------+-----+---------+----------------+ 5 rows in set (0.02 sec)
Now we need to create a MySQL stored procedure to add items to the tbl_blog table:
USE `FlaskBlogApp`; DROP procedure IF EXISTS `FlaskBlogApp`.`sp_addBlog`; DELIMITER $$ USE `FlaskBlogApp`$$ CREATE DEFINER=`root`@`localhost` PROCEDURE `sp_addBlog`( IN p_title varchar(45), IN p_description varchar(1000), IN p_user_id bigint ) BEGIN insert into tbl_blog( blog_title, blog_description, blog_user_id, blog_date ) values ( p_title, p_description, p_user_id, NOW() ); END$$ DELIMITER ;
Since we'll be posting data to the method called addBlog(), we have explicitly declared it in the defined route as shown below:
@app.route('/addBlog',methods=['POST']) def addBlog():
When a call is made to the addBlog() method, we may want to validate it's an authenticity by checking if the session variable user exists:
if session.get('user'):
If it's a valid session, we'll read the posted 'title' and 'description':
if session.get('user'): _title = request.form['inputTitle'] _description = request.form['inputDescription'] _user = session.get('user')
Now that we have the required input values, we want to open a MySQL connection and call the stored procedure sp_addBlog:
conn = mysql.connect() cursor = conn.cursor() cursor.callproc('sp_addBlog',(_title,_description,_user)) data = cursor.fetchall()
Once the stored procedure is executed, we need to commit the changes to the database:
if len(data) is 0: conn.commit() return redirect('/userHome') else: return render_template('error.html',error = 'An error occurred!')
Now we can add a blog post:
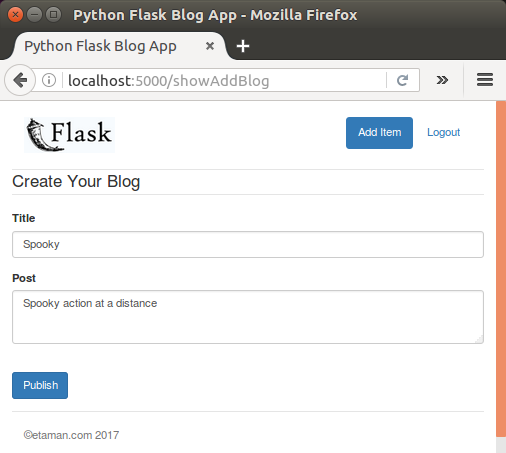
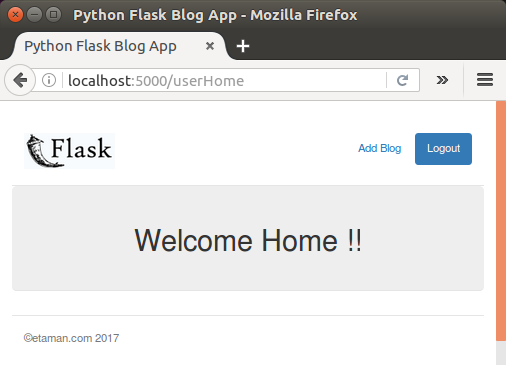
Here are the output from the server console and we can see the process from the sign-in to post:
(venv1) k@laptop:~/MySites/etaman/FlaskApp/p3$ python app.py * Running on http://127.0.0.1:5000/ (Press CTRL+C to quit) "GET / HTTP/1.1" 200 - "GET /showSignin HTTP/1.1" 200 - "POST /validateLogin HTTP/1.1" 302 - "GET /userHome HTTP/1.1" 200 - "GET /showAddBlog HTTP/1.1" 200 - "POST /addBlog HTTP/1.1" 302 - "GET /userHome HTTP/1.1" 200 -
We can check if our blog is in the table:
mysql> select * from tbl_blog; +---------+------------+-----------------------------+--------------+---------------------+ | blog_id | blog_title | blog_description | blog_user_id | blog_date | +---------+------------+-----------------------------+--------------+---------------------+ | 3 | Spooky | Spooky action at a distance | 1 | 2016-12-03 19:29:35 | +---------+------------+-----------------------------+--------------+---------------------+ 1 row in set (0.00 sec)
We need to create a MySQL stored procedure to retrieve the blog created by a user. It will take the user ID as a parameter and return a data set of blogs created by the particular user ID:
USE `FlaskBlogApp`; DROP procedure IF EXISTS `sp_GetBlogByUser`; DELIMITER $$ USE `FlaskBlogApp`$$ CREATE PROCEDURE `sp_GetBlogByUser` ( IN p_user_id bigint ) BEGIN select * from tbl_blog where blog_user_id = p_user_id; END$$ DELIMITER ;
We can check the stored procedures created so far:
mysql> SHOW PROCEDURE STATUS WHERE db = 'FlaskBlogApp'; +--------------+------------------+-----------+----------------+---------------------+---------------------+---------------+---------+----------------------+----------------------+--------------------+ | Db | Name | Type | Definer | Modified | Created | Security_type | Comment | character_set_client | collation_connection | Database Collation | +--------------+------------------+-----------+----------------+---------------------+---------------------+---------------+---------+----------------------+----------------------+--------------------+ | FlaskBlogApp | sp_addBlog | PROCEDURE | root@localhost | 2016-12-03 17:00:03 | 2016-12-03 17:00:03 | DEFINER | | utf8 | utf8_general_ci | latin1_swedish_ci | | FlaskBlogApp | sp_createUser | PROCEDURE | root@localhost | 2016-12-02 21:50:34 | 2016-12-02 21:50:34 | DEFINER | | utf8 | utf8_general_ci | latin1_swedish_ci | | FlaskBlogApp | sp_GetBlogByUser | PROCEDURE | root@localhost | 2016-12-03 19:58:11 | 2016-12-03 19:58:11 | DEFINER | | utf8 | utf8_general_ci | latin1_swedish_ci | | FlaskBlogApp | sp_validateLogin | PROCEDURE | root@localhost | 2016-12-03 11:09:38 | 2016-12-03 11:09:38 | DEFINER | | utf8 | utf8_general_ci | latin1_swedish_ci | +--------------+------------------+-----------+----------------+---------------------+---------------------+---------------+---------+----------------------+----------------------+--------------------+ 4 rows in set (0.15 sec)
Now we need to create a method which will call the sp_GetBlogByUser stored procedure to get the posts created by a user. Let's add a getBlog() method in app.py:
@app.route('/getBlog') def getBlog(): try: if session.get('user'): _user = session.get('user') con = mysql.connect() cursor = con.cursor() cursor.callproc('sp_GetBlogByUser',(_user,)) blogs = cursor.fetchall() blogs_dict = [] for blog in blogs: blog_dict = { 'Id': blog[0], 'Title': blog[1], 'Description': blog[2], 'Date': blog[4]} blogs_dict.append(blog_dict) return json.dumps(blogs_dict) else: return render_template('error.html', error = 'Unauthorized Access') except Exception as e: return render_template('error.html', error = str(e))
As we can see from the code, this method can only be called with valid user session:
@app.route('/getBlog') def getBlog(): try: if session.get('user'): ... else: ... except Exception as e: ...
If it is a valid user session, we create a connection to the MySQL database and call the stored procedure sp_GetBlogByUser:
if session.get('user'): _user = session.get('user') con = mysql.connect() cursor = con.cursor() cursor.callproc('sp_GetBlogByUser',(_user,)) blogs = cursor.fetchall()
After fetching data from MySQL, we'll parse the data and convert it into a dictionary so that it's easy to return as JSON:
if session.get('user'): ... blogs_dict = [] for blog in blogs: blog_dict = { 'Id': blog[0], 'Title': blog[1], 'Description': blog[2], 'Date': blog[4]} blogs_dict.append(blog_dict) return json.dumps(blogs_dict)
After converting the data into a dictionary we convert the data into JSON and return.
Once the user's home page is loaded, we call the getBlog() method using jQuery AJAX and bind the received data into our HTML. Let's add the following jQuery AJAX script to userHome.html:
<script> $(function() { $.ajax({ url: '/getBlog', type: 'GET', success: function(res) { console.log(res); }, error: function(error) { console.log(error); } }); }); </script>
Let's restart the server:
(venv1) k@laptop:~/MySites/etaman/FlaskApp/p3$ python app.py * Running on http://127.0.0.1:5000/ (Press CTRL+C to quit)
Once logged in with a valid email address and password, check the browser console and we should have the blog list retrieved from the database as shown:
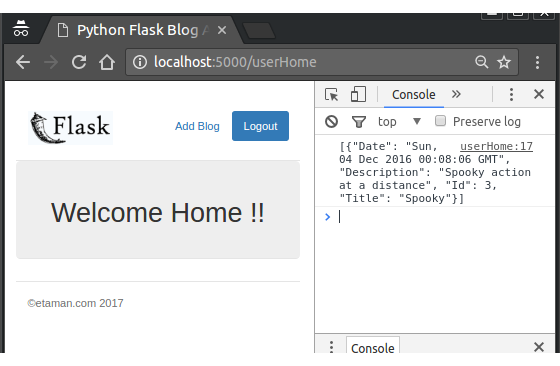
Now, we may want to iterate over the JSON data and bind it into the HTML. We'll be using bootstrap list-group to display our wish list items. Here is the userHome.html:
<!DOCTYPE html> <html lang="en"> <head> <title>Python Flask Blog App</title> <link href="//getbootstrap.com/dist/css/bootstrap.min.css" rel="stylesheet"> <link href="//getbootstrap.com/examples/jumbotron-narrow/jumbotron-narrow.css" rel="stylesheet"> <link href="/static/css/signup.css" rel="stylesheet"> <script src="/static/js/jquery-3.1.1.js"></script> <!--<script> $(function() { $.ajax({ url: '/getBlog', type: 'GET', success: function(res) { console.log(res); }, error: function(error) { console.log(error); } }); }); </script> --> <script> $(function(){ $.ajax({ url : '/getBlog', type : 'GET', success: function(res){ var div = $('<div>') .attr('class', 'list-group') .append($('<a>') .attr('class', 'list-group-item active') .append($('<h4>') .attr('class', 'list-group-item-heading'), $('<p>') .attr('class', 'list-group-item-text'))); var blogObj = JSON.parse(res); var blog = ''; $.each(blogObj,function(index, value){ blog = $(div).clone(); $(blog).find('h4').text(value.Title); $(blog).find('p').text(value.Description); $('.jumbotron').append(blog); }); }, error: function(error){ console.log(error); } }); }); </script> </head> <body> <div class="container"> <div class="header"> <nav> <ul class="nav nav-pills pull-right"> <li role="presentation"><a href="/showAddBlog">Add Blog</a></li> <li role="presentation" class="active"><a href="/logout">Logout</a></li> </ul> </nav> <img src="/static/images/Flask_Icon.png" alt="Flask_Icon.png"/ > </div> <div class="jumbotron"> <h1>Welcome Home !!</h1> </div> <footer class="footer"> <p>©etaman.com 2017</p> </footer> </div> </body> </html>
So, when a successful login, the user will have the following page that lists blog posts:
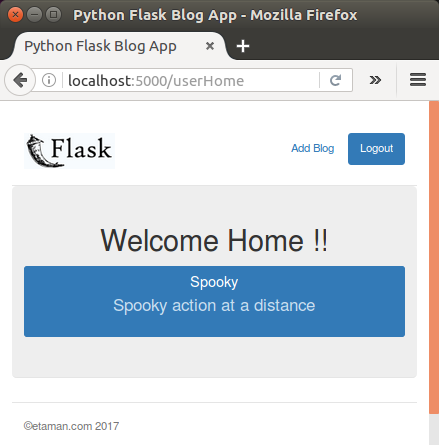
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization