Flask app 5 - Word counts app with AngularJS front-end updates and submit error handling
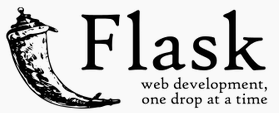
In the previous section (Flask app 4 : word counts with AngularJS polling the back-end), we setup the back-end polling and implemented basic AngularJS front-end.
In this tutorial, we'll update AngularJS for more user friendly front-end.
Here are the items that we need to do:
- We'll disable the submit button to prevent users from continually clicking while they are waiting for the submitted site to be counted.
- Then, while the application is counting the words, we'll add a display throbber/loading spinner where the word count list will go to show the user that there is activity happening in the back-end.
- Lastly, we'll display an error if the domain is unable to be reached.
Github source : akadrone-flask
Change the button in templates/index.html:
{% raw %} <button type="submit" class="btn btn-primary" ng-disabled="loading">{{ submitButtonText }}</button> {% endraw %}
Here, we added an ng-disabled directive and attached that to loading. This will disable the button when loading evaluates to true.
Then, we added a variable to display to the user called submitButtonText. This way we'll be able to change the text from Submit to Loading... so that the user knows what's going on.
We then wrapped the button in {% raw %} and {% endraw %} so that Jinja knows to evaluate this as raw HTML. If we don't do this, Flask will try to evaluate the {{ submitButtonText }} as a Jinja variable and Angular won't get a chance to evaluate it.
To make it work, we also need to change some lines in static/main.js. At the top of the WordcountController in main.js:
(function () { 'use strict'; angular.module('WordcountApp', []) .controller('WordcountController', ['$scope', '$log', '$http', '$timeout', function($scope, $log, $http, $timeout) { $scope.submitButtonText = 'Submit'; $scope.loading = false; $scope.getResults = function() {
This sets the initial value of loading to false so that the button will not be disabled.
Another modification:
(function () { 'use strict'; angular.module('WordcountApp', []) .controller('WordcountController', ['$scope', '$log', '$http', '$timeout', function($scope, $log, $http, $timeout) { $scope.submitButtonText = 'Submit'; $scope.loading = false; $scope.getResults = function() { $log.log('test'); // get the URL from the input var userInput = $scope.url; // fire the API request $http.post('/start', {'url': userInput}). success(function(results) { $log.log(results); getWordCount(results); $scope.wordcounts = null; $scope.loading = true; $scope.submitButtonText = 'Loading...'; }). error(function(error) { $log.log(error); }); };
In the code, we set:
- wordcounts to null so that old values get cleared out.
- loading to true so that the loading button will be disabled via the ng-disabled directive we added to the HTML.
- submitButtonText to Loading... so that the user knows why the button is disabled.
The relevant change should be made for poller(). In other words, when the result is successful, we set loading back to false so that the button is enabled again and changed the button text back to Submit so the user knows they can submit a new URL.
function getWordCount(jobID) { var timeout = ''; var poller = function() { // fire another request $http.get('/results/'+jobID). success(function(data, status, headers, config) { if(status === 202) { $log.log(data, status); } else if (status === 200){ $log.log(data); $scope.loading = false; $scope.submitButtonText = "Submit"; $scope.wordcounts = data; $timeout.cancel(timeout); return false; } // continue to call the poller() function every 2 seconds // until the timeout is cancelled timeout = $timeout(poller, 2000); }); }; poller(); }
Spinner let the user know what's going on while the code is counting words.
<!DOCTYPE html> <html ng-app="WordcountApp"> <head> <title>Wordcount</title> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="//netdna.bootstrapcdn.com/bootstrap/3.1.1/css/bootstrap.min.css" rel="stylesheet" media="screen"> <style> .container { max-width: 1000px; } </style> </head> <body ng-controller="WordcountController"> <div class="container"> <div class="row"> <div class="col-sm-5 col-sm-offset-1"> <h1>Wordcount</h1> <br> <form role="form" ng-submit="getResults()"> <div class="form-group"> <input type="text" name="url" class="form-control" id="url-box" placeholder="Enter URL..." style="max-width: 300px;" ng-model="url" required> </div> {% raw %} <button type="submit" class="btn btn-primary" ng-disabled="loading">{{ submitButtonText }}</button> {% endraw %} </form> <br> {% for error in errors %} <h4>{{ error }}</h4> {% endfor %} <br> </div> <div class="col-sm-5 col-sm-offset-1"> <h2>Frequencies</h2> <br> <div id="results"> <table class="table table-striped"> <thead> <tr> <th>Word</th> <th>Count</th> </tr> </thead> <tbody> {% raw %} <tr ng-repeat="(key, val) in wordcounts"> <td>{{key}}</td> <td>{{val}}</td> </tr> {% endraw %} </tbody> </table> </div> </div> <img class="col-sm-3 col-sm-offset-4" src="{{ url_for('static', filename='spinner.gif') }}" ng-show="loading"> </div> </div> <br><br> <script src="//code.jquery.com/jquery-1.11.0.min.js"></script> <script src="//netdna.bootstrapcdn.com/bootstrap/3.1.1/js/bootstrap.min.js"></script> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.4.9/angular.min.js"></script> <script src="{{ url_for('static', filename='main.js') }}"></script> </body> </html>
Note that we need to put spinner.gif into static directory.
We can also see that ng-show is attached to loading.
We're using Bootstrap's alert class to show a warning dialog if the user submits a bad URL. By using Angular's ng-show directive we can only display the dialog when urlerror is true.
templates/index.html:
<!DOCTYPE html> <html ng-app="WordcountApp"> <head> <title>Wordcount</title> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="//netdna.bootstrapcdn.com/bootstrap/3.1.1/css/bootstrap.min.css" rel="stylesheet" media="screen"> <style> .container { max-width: 1000px; } </style> </head> <body ng-controller="WordcountController"> <div class="container"> <div class="row"> <div class="col-sm-5 col-sm-offset-1"> <h1>Wordcount</h1> <br> <form role="form" ng-submit="getResults()"> <div class="form-group"> <input type="text" name="url" class="form-control" id="url-box" placeholder="Enter URL..." style="max-width: 300px;" ng-model="url" required> </div> {% raw %} <button type="submit" class="btn btn-primary" ng-disabled="loading">{{ submitButtonText }}</button> {% endraw %} </form> <br> <div class="alert alert-danger" role="alert" ng-show='urlError'> <span class="glyphicon glyphicon-exclamation-sign" aria-hidden="true"></span> <span class="sr-only">Error:</span> <span>There was an error submitting your URL.<br> Please check to make sure it is valid before trying again.</span> </div> <br> </div> <div class="col-sm-5 col-sm-offset-1"> <h2>Frequencies</h2> <br> <div id="results"> <table class="table table-striped"> <thead> <tr> <th>Word</th> <th>Count</th> </tr> </thead> <tbody> {% raw %} <tr ng-repeat="(key, val) in wordcounts"> <td>{{key}}</td> <td>{{val}}</td> </tr> {% endraw %} </tbody> </table> </div> </div> <img class="col-sm-3 col-sm-offset-4" src="{{ url_for('static', filename='spinner.gif') }}" ng-show="loading"> </div> </div> <br><br> <script src="//code.jquery.com/jquery-1.11.0.min.js"></script> <script src="//netdna.bootstrapcdn.com/bootstrap/3.1.1/js/bootstrap.min.js"></script> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.4.9/angular.min.js"></script> <script src="{{ url_for('static', filename='main.js') }}"></script> </body> </html>
static/main.js:
(function () { 'use strict'; angular.module('WordcountApp', []) .controller('WordcountController', ['$scope', '$log', '$http', '$timeout', function($scope, $log, $http, $timeout) { $scope.submitButtonText = 'Submit'; $scope.loading = false; $scope.urlerror = false; $scope.getResults = function() { $log.log('test'); // get the URL from the input var userInput = $scope.url; // fire the API request $http.post('/start', {'url': userInput}). success(function(results) { $log.log(results); getWordCount(results); $scope.wordcounts = null; $scope.loading = true; $scope.submitButtonText = 'Loading...'; $scope.urlerror = false; }). error(function(error) { $log.log(error); }); }; function getWordCount(jobID) { var timeout = ''; var poller = function() { // fire another request $http.get('/results/'+jobID). success(function(data, status, headers, config) { if(status === 202) { $log.log(data, status); } else if (status === 200){ $log.log(data); $scope.loading = false; $scope.submitButtonText = "Submit"; $scope.wordcounts = data; $timeout.cancel(timeout); return false; } // continue to call the poller() function every 2 seconds // until the timeout is cancelled timeout = $timeout(poller, 2000); }). error(function(error) { $log.log(error); $scope.loading = false; $scope.submitButtonText = "Submit"; $scope.urlerror = true; }); }; poller(); } }]); }());
We've made the user interface more user friendly so that the user knows what is happening while we are running the word count functionality behind the scenes.
Let's test out our new implementations.
Loading...
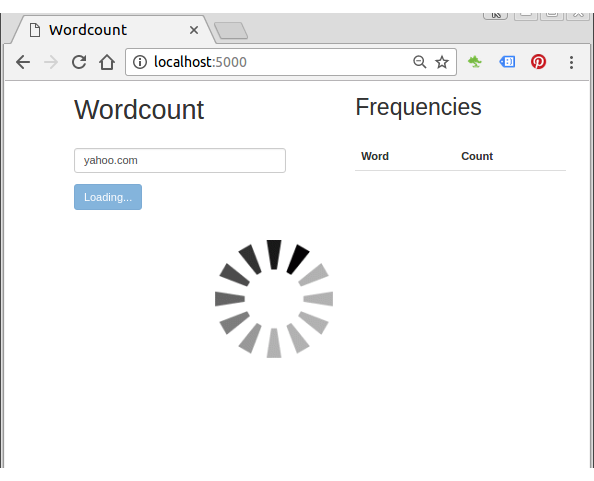
Loading finished:
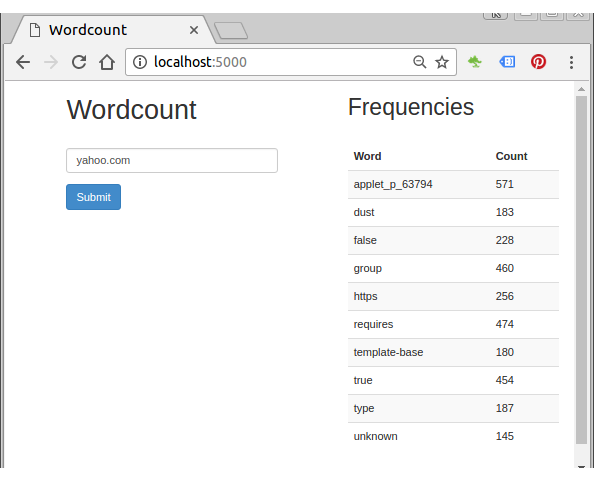
If we want to access the page with port 80, we can setup proxy. For Apache, it looks like this:
<VirtualHost *:80> ServerName www.akadrone.example.com ServerAlias akadrone.example.com ProxyPreserveHost On ProxyPass / http://127.0.0.1:5000/ #ProxyPassReverse / http://127.0.0.1:5000/ ErrorLog /var/www/akadrone.com/logs/error.log CustomLog /var/www/akadrone.com/logs/access.log combined LogLevel warn ServerSignature Off </VirtualHost>
Run gunicorn.sh:
$ ./gunicorn_aka.sh
where the file (gunicorn_aka.sh) should look like this:
source /home/k/MySites/akadrone/venv/bin/activate python /home/k/MySites/akadrone/worker.py & exec gunicorn -w 4 -b 127.0.0.1:5000 aka:app
As we can see from the script, we're running two programs: worker.py and our app (aka.py via gunicorn).
Then, we can access our app either with:
localhost:5000
or
akadrone.example.com:80
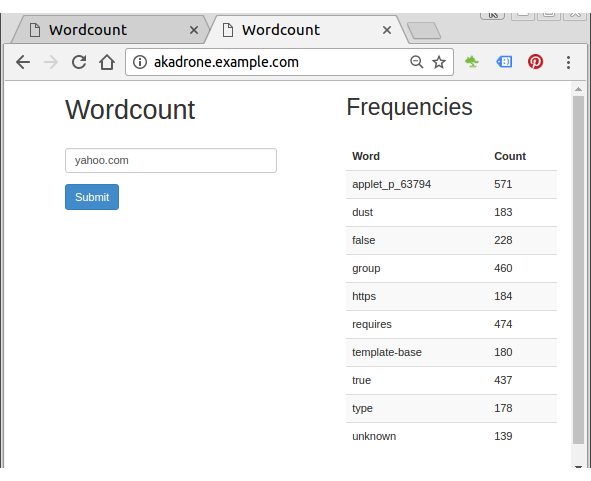
To run in background, we can use pm2:
$ pm2 start gunicorn_aka.sh
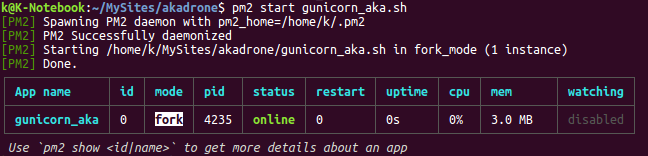
Here are the screen shots from akadrone.com deployed on CentOS 7 server:
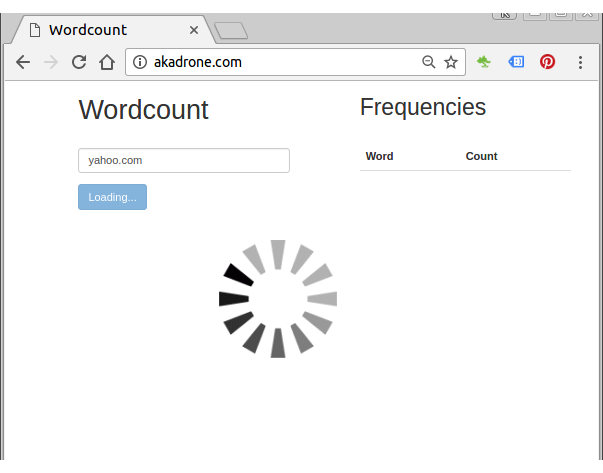
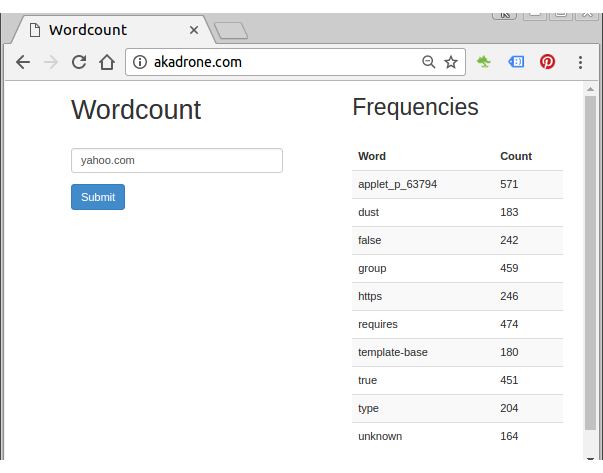
We used pm2:
(venv)[sfvue@sf akadrone]$ pm2 start ./gunicorn_aka.sh
with gunicorn.sh:
source /home/k/MySites/akadrone/venv/bin/activate /home/sfvue/MySites/akadrone/venv/bin/python /home/sfvue/MySites/akadrone/worker.py & /home/sfvue/MySites/akadrone/venv/bin/gunicorn -w 1 -b 127.0.0.1:5000 aka:app
Apache proxy config (/etc/httpd/sites-available/akadrone.com.conf):
<VirtualHost *:80> ServerName www.akadrone.com ServerAlias akadrone.com ProxyPreserveHost On ProxyPass / http://127.0.0.1:5000/ #ProxyPassReverse / http://127.0.0.1:5000/ ErrorLog /var/www/akadrone.com/logs/error.log CustomLog /var/www/akadrone.com/logs/access.log combined LogLevel warn ServerSignature Off </VirtualHost>
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization