Flask blog app tutorial 7 : Like button
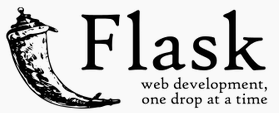
In the previous part of this series, we populated the dashboard page of our application with the blog posts created by different users. We also attached a like button to each post so that a user could like a particular post.
In this part of the series, we'll add toggle feature for the like/unlike display. Also, we'll show the total number of likes received by a particular post.
Here are the files we'll be using in this tutorial part-7:
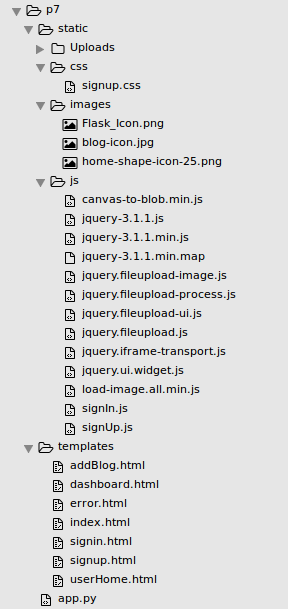
They are available from FlaskApp/p7
We'll start by implementing a feature to show the total number of counts a particular blog post. When a new blog post gets added, we'll make an entry into the tbl_likes table. So, we need to modify the MySQL stored procedure sp_addBlog to add an entry into the tbl_likes table.
In the procedure, sp_addBlog, after inserting the blog post into the tbl_blog table, we fetched the last inserted ID and inserted the data into tbl_likes table:
USE `FlaskBlogApp`; DROP procedure IF EXISTS `sp_addBlog`; DELIMITER $$ CREATE DEFINER=`root`@`localhost` PROCEDURE `sp_addBlog`( IN p_title varchar(45), IN p_description varchar(1000), IN p_user_id bigint, IN p_file_path varchar(200), IN p_is_private int, IN p_is_done int ) BEGIN insert into tbl_blog( blog_title, blog_description, blog_user_id, blog_date, blog_file_path, blog_private, blog_accomplished ) values ( p_title, p_description, p_user_id, NOW(), p_file_path, p_is_private, p_is_done ); SET @last_id = LAST_INSERT_ID(); insert into tbl_likes( blog_id, user_id, blog_like ) values( @last_id, p_user_id, 0 ); END$$ DELIMITER ;
Now, we need to modify the sp_GetAllBlogs stored procedure to include the number of likes each blog post. We'll make use of a MySQL function to get the total number of blog posts. So, let's create a function called getSum which will take the blog post ID and return the total number of likes.
USE `FlaskBlogApp`; DROP FUNCTION IF EXISTS getSum; DELIMITER $$ CREATE DEFINER=`root`@`localhost` FUNCTION `getSum`( p_blog_id int ) RETURNS int(11) BEGIN select sum(blog_like) into @sm from tbl_likes where blog_id = p_blog_id; RETURN @sm; END$$ DELIMITER ;
Now, call the above MySQL function called getSum in the stored procedure sp_GetAllBlogs to get the total number of likes for each blog post:
USE `FlaskBlogApp`; DROP procedure IF EXISTS `sp_GetAllBlogs`; DELIMITER $$ CREATE DEFINER=`root`@`localhost` PROCEDURE `sp_GetAllBlogs`() BEGIN select blog_id,blog_title,blog_description,blog_file_path,getSum(blog_id) from tbl_blog where blog_private = 0; END$$ DELIMITER ;
If we run the MySQL script on mysql> :
mysql> select blog_id,blog_title,getSum(blog_id) from tbl_blog; +---------+-------------------------------------------+-----------------+ | blog_id | blog_title | getSum(blog_id) | +---------+-------------------------------------------+-----------------+ | 3 | Spooky | 1 | | 4 | Curiosity has its own reason for existing | NULL | | 5 | On certainty ??? | 1 | | 7 | Test | 1 | | 8 | test 2 | NULL | | 9 | test 3 | NULL | | 10 | test 4 | 1 | | 12 | test6 | NULL | | 14 | Canada | 1 | +---------+-------------------------------------------+-----------------+ 9 rows in set (0.00 sec)
Modify the getAllBlogs() method to include the like count. While iterating the result returned from the MySQL stored procedure, include the like field as shown below:
@app.route('/getAllBlogs') def getAllBlogs(): try: if session.get('user'): conn = mysql.connect() cursor = conn.cursor() cursor.callproc('sp_GetAllBlogs') result = cursor.fetchall() blogs_dict = [] for blog in result: blog_dict = { 'Id': blog[0], 'Title': blog[1], 'Description': blog[2], 'FilePath': blog[3], 'Like':blog[4]} bloges_dict.append(blog_dict) return json.dumps(blogs_dict) else: return render_template('error.html', error = 'Unauthorized Access') except Exception as e: return render_template('error.html',error = str(e))
hasLiked:
USE `FlaskBlogApp`; DROP Function IF EXISTS `hasLiked`; DELIMITER $$ CREATE DEFINER=`root`@`localhost` FUNCTION `hasLiked`( p_blog int, p_user int ) RETURNS int(11) BEGIN select blog_like into @myval from tbl_likes where blog_id = p_blog and user_id = p_user; RETURN @myval; END$$ DELIMITER ;
USE `FlaskBlogApp`; DROP procedure IF EXISTS `sp_GetAllBlogs`; DELIMITER $$ CREATE DEFINER=`root`@`localhost` PROCEDURE `sp_GetAllBlogs`( p_user int ) BEGIN select blog_id,blog_title,blog_description,blog_file_path,getSum(blog_id),hasLiked(blog_id,p_user) from tbl_blog where blog_private = 0; END$$ DELIMITER ;
sp_AddUpdateLikes:
USE `FlaskBlogApp`; DROP procedure IF EXISTS `sp_AddUpdateLikes`; DELIMITER $$ CREATE DEFINER=`root`@`localhost` PROCEDURE `sp_AddUpdateLikes`( p_blog_id int, p_user_id int, p_like int ) BEGIN if (select exists (select 1 from tbl_likes where blog_id = p_blog_id and user_id = p_user_id)) then select blog_like into @currentVal from tbl_likes where blog_id = p_blog_id and user_id = p_user_id; if @currentVal = 0 then update tbl_likes set blog_like = 1 where blog_id = p_blog_id and user_id = p_user_id; else update tbl_likes set blog_like = 0 where blog_id = p_blog_id and user_id = p_user_id; end if; else insert into tbl_likes( blog_id, user_id, blog_like ) values( p_blog_id, p_user_id, p_like ); end if; END$$ DELIMITER ;
sp_getLikeStatus:
USE `FlaskBlogApp`; DROP procedure IF EXISTS `sp_getLikeStatus`; DELIMITER $$ CREATE DEFINER=`root`@`localhost` PROCEDURE `sp_getLikeStatus`( IN p_blog_id int, IN p_user_id int ) BEGIN select getSum(p_blog_id),hasLiked(p_blog_id,p_user_id); END$$ DELIMITER ;
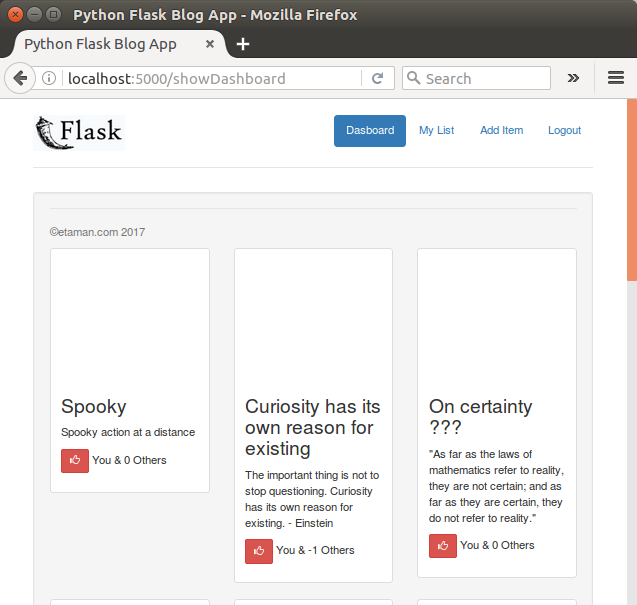
Here is the final version of app.py:
from flask import Flask, render_template, json, request, redirect, session, jsonify, url_for from flaskext.mysql import MySQL from werkzeug import generate_password_hash, check_password_hash import os import uuid mysql = MySQL() app = Flask(__name__) app.secret_key = 'spooky action at a distance-Einstein' # MySQL configurations app.config['MYSQL_DATABASE_USER'] = 'khong' app.config['MYSQL_DATABASE_PASSWORD'] = 'khong' app.config['MYSQL_DATABASE_DB'] = 'FlaskBlogApp' app.config['MYSQL_DATABASE_HOST'] = 'localhost' app.config['UPLOAD_FOLDER'] = 'static/Uploads' mysql.init_app(app) # Default setting pageLimit = 5 @app.route('/') def main(): return render_template('index.html') @app.route('/upload', methods=['GET', 'POST']) def upload(): if request.method == 'POST': file = request.files['file'] extension = os.path.splitext(file.filename)[1] f_name = str(uuid.uuid4()) + extension file.save(os.path.join(app.config['UPLOAD_FOLDER'], f_name)) return json.dumps({'filename':f_name}) @app.route('/showSignUp') def showSignUp(): return render_template('signup.html') @app.route('/showAddBlog') def showAddBlog(): return render_template('addBlog.html') @app.route('/addUpdateLike',methods=['POST']) def addUpdateLike(): try: if session.get('user'): _blogId = request.form['blog'] _like = request.form['like'] _user = session.get('user') conn = mysql.connect() cursor = conn.cursor() cursor.callproc('sp_AddUpdateLikes',(_blogId,_user,_like)) data = cursor.fetchall() if len(data) is 0: conn.commit() cursor.close() conn.close() conn = mysql.connect() cursor = conn.cursor() cursor.callproc('sp_getLikeStatus',(_blogId,_user)) result = cursor.fetchall() return json.dumps({'status':'OK','total':result[0][0],'likeStatus':result[0][1]}) else: return render_template('error.html',error = 'An error occurred!') else: return render_template('error.html',error = 'Unauthorized Access') except Exception as e: return render_template('error.html',error = str(e)) finally: cursor.close() conn.close() @app.route('/getAllBlogs') def getAllBlogs(): try: if session.get('user'): _user = session.get('user') conn = mysql.connect() cursor = conn.cursor() cursor.callproc('sp_GetAllBlogs',(_user,)) result = cursor.fetchall() blogs_dict = [] for blog in result: blog_dict = { 'Id': blog[0], 'Title': blog[1], 'Description': blog[2], 'FilePath': blog[3], 'Like':blog[4], 'HasLiked':blog[5]} blogs_dict.append(blog_dict) return json.dumps(blogs_dict) else: return render_template('error.html', error = 'Unauthorized Access') except Exception as e: return render_template('error.html',error = str(e)) @app.route('/showDashboard') def showDashboard(): return render_template('dashboard.html') @app.route('/showSignin') def showSignin(): if session.get('user'): return render_template('userHome.html') else: return render_template('signin.html') @app.route('/userHome') def userHome(): if session.get('user'): return render_template('userHome.html') else: return render_template('error.html',error = 'Unauthorized Access') @app.route('/logout') def logout(): session.pop('user',None) return redirect('/') @app.route('/deleteBlog',methods=['POST']) def deleteBlog(): try: if session.get('user'): _id = request.form['id'] _user = session.get('user') conn = mysql.connect() cursor = conn.cursor() cursor.callproc('sp_deleteBlog',(_id,_user)) result = cursor.fetchall() if len(result) is 0: conn.commit() return json.dumps({'status':'OK'}) else: return json.dumps({'status':'An Error occured'}) else: return render_template('error.html',error = 'Unauthorized Access') except Exception as e: return json.dumps({'status':str(e)}) finally: cursor.close() conn.close() @app.route('/getBlogById',methods=['POST']) def getBlogById(): try: if session.get('user'): _id = request.form['id'] _user = session.get('user') conn = mysql.connect() cursor = conn.cursor() cursor.callproc('sp_GetBlogById',(_id,_user)) result = cursor.fetchall() blog = [] blog.append({'Id':result[0][0],'Title':result[0][1],'Description':result[0][2],'FilePath':result[0][3],'Private':result[0][4],'Done':result[0][5]}) return json.dumps(blog) else: return render_template('error.html', error = 'Unauthorized Access') except Exception as e: return render_template('error.html',error = str(e)) @app.route('/getBlog',methods=['POST']) def getBlog(): try: if session.get('user'): _user = session.get('user') _limit = pageLimit _offset = request.form['offset'] _total_records = 0 con = mysql.connect() cursor = con.cursor() cursor.callproc('sp_GetBlogByUser',(_user,_limit,_offset,_total_records)) blogs = cursor.fetchall() cursor.close() cursor = con.cursor() cursor.execute('SELECT @_sp_GetBlogByUser_3'); outParam = cursor.fetchall() response = [] blogs_dict = [] for blog in blogs: blog_dict = { 'Id': blog[0], 'Title': blog[1], 'Description': blog[2], 'Date': blog[4]} blogs_dict.append(blog_dict) response.append(blogs_dict) response.append({'total':outParam[0][0]}) return json.dumps(response) else: return render_template('error.html', error = 'Unauthorized Access') except Exception as e: return render_template('error.html', error = str(e)) @app.route('/addBlog',methods=['POST']) def addBlog(): try: if session.get('user'): _title = request.form['inputTitle'] _description = request.form['inputDescription'] _user = session.get('user') if request.form.get('filePath') is None: _filePath = '' else: _filePath = request.form.get('filePath') if request.form.get('private') is None: _private = 0 else: _private = 1 if request.form.get('done') is None: _done = 0 else: _done = 1 conn = mysql.connect() cursor = conn.cursor() cursor.callproc('sp_addBlog',(_title,_description,_user,_filePath,_private,_done)) data = cursor.fetchall() if len(data) is 0: conn.commit() return redirect('/userHome') else: return render_template('error.html',error = 'An error occurred!') else: return render_template('error.html',error = 'Unauthorized Access') except Exception as e: return render_template('error.html',error = str(e)) finally: cursor.close() conn.close() @app.route('/updateBlog', methods=['POST']) def updateBlog(): try: if session.get('user'): _user = session.get('user') _title = request.form['title'] _description = request.form['description'] _blog_id = request.form['id'] _filePath = request.form['filePath'] _isPrivate = request.form['isPrivate'] _isDone = request.form['isDone'] conn = mysql.connect() cursor = conn.cursor() cursor.callproc('sp_updateBlog',(_title,_description,_blog_id,_user,_filePath,_isPrivate,_isDone)) data = cursor.fetchall() if len(data) is 0: conn.commit() return json.dumps({'status':'OK'}) else: return json.dumps({'status':'ERROR'}) except Exception as e: return json.dumps({'status':'Unauthorized access'}) finally: cursor.close() conn.close() @app.route('/validateLogin',methods=['POST']) def validateLogin(): try: _username = request.form['inputEmail'] _password = request.form['inputPassword'] # connect to mysql con = mysql.connect() cursor = con.cursor() cursor.callproc('sp_validateLogin',(_username,)) data = cursor.fetchall() if len(data) > 0: if check_password_hash(str(data[0][3]),_password): session['user'] = data[0][0] return redirect('/showDashboard') else: return render_template('error.html',error = 'Wrong Email address or Password.') else: return render_template('error.html',error = 'Wrong Email address or Password.') except Exception as e: return render_template('error.html',error = str(e)) finally: cursor.close() con.close() @app.route('/signUp',methods=['POST','GET']) def signUp(): try: _name = request.form['inputName'] _email = request.form['inputEmail'] _password = request.form['inputPassword'] # validate the received values if _name and _email and _password: # All Good, let's call MySQL conn = mysql.connect() cursor = conn.cursor() _hashed_password = generate_password_hash(_password) cursor.callproc('sp_createUser',(_name,_email,_hashed_password)) data = cursor.fetchall() if len(data) is 0: conn.commit() return json.dumps({'message':'User created successfully !'}) else: return json.dumps({'error':str(data[0])}) else: return json.dumps({'html':'<span>Enter the required fields</span>'}) except Exception as e: return json.dumps({'error':str(e)}) finally: cursor.close() conn.close() if __name__ == "__main__": app.run(port=5000)
THe final version of dashboard.html looks like this:
<!DOCTYPE html> <html lang="en"> <head> <title>Python Flask Blog App</title> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.2/css/bootstrap.min.css"> <link href="//getbootstrap.com/examples/jumbotron-narrow/jumbotron-narrow.css" rel="stylesheet"> <script src="/static/js/jquery-3.1.1.js"></script> <script> $(function(){ $.ajax({ url: '/getAllBlogs', type: 'GET', success:function(response) { console.log(response); var data = JSON.parse(response); var itemsPerRow = 0; var div = $('<div>').attr('class','row'); for(var i=0;i<data.length;i++){ console.log(data[i].Title); if(itemsPerRow<3){ console.log(i); if(i==data.length-1){ div.append(CreateThumb(data[i].Id,data[i].Title,data[i].Description,data[i].FilePath,data[i].Like,data[i].HasLiked)); $('.well').append(div); } else{ div.append(CreateThumb(data[i].Id,data[i].Title,data[i].Description,data[i].FilePath,data[i].Like,data[i].HasLiked)); itemsPerRow++; } } else{ $('.well').append(div); div = $('<div>').attr('class','row'); div.append(CreateThumb(data[i].Id,data[i].Title,data[i].Description,data[i].FilePath,data[i].Like,data[i].HasLiked)); if(i==data.length-1){ $('.well').append(div); } itemsPerRow = 0; } } }, error:function(error){ console.log(error); } }); $(document).on('click','[id^="btn_"]',function(){ var spId = $(this).attr('id').split('_')[1]; //alert($(this).attr('id').split('_')[1]); $.ajax({ url: '/addUpdateLike', method: 'POST', data: {blog:$(this).attr('id').split('_')[1],like:1}, success: function(response){ var obj = JSON.parse(response); if(obj.likeStatus=="1"){ console.log(obj.likeStatus); $('#span_'+spId).html(' You & '+ (Number(obj.total)-1) + ' Others'); } else{ $('#span_'+spId).html(' '+ obj.total + ' like(s)'); } }, error: function(error){ console.log(error); } }); }); }) function CreateThumb(id,title,desc,filepath,like,hasLiked){ var mainDiv = $('<div>').attr('class','col-sm-4 col-md-4'); var thumbNail = $('<div>').attr('class','thumbnail'); var img = $('<img>').attr({'src':filepath,'data-holder-rendered':true,'style':'height: 150px; width: 150px; display: block'}); var caption = $('<div>').attr('class','caption'); var title = $('<h3>').text(title); var desc = $('<p>').text(desc); var p = $('<p>'); var btn = $('<button>').attr({'id':'btn_'+id,'type':'button','class':'btn btn-danger btn-sm'}); var span = $('<span>').attr({'class':'glyphicon glyphicon-thumbs-up','aria-hidden':'true'}); var likeSpan = $('<span>').attr({'aria-hidden':'true','id':'span_'+id}); if(hasLiked == "1"){ likeSpan.html(' You & '+ (Number(like)-1) + ' Others'); } else{ likeSpan.html(' '+ like + ' like(s)'); } p.append(btn.append(span)); p.append(likeSpan); caption.append(title); caption.append(desc); caption.append(p); thumbNail.append(img); thumbNail.append(caption); mainDiv.append(thumbNail); return mainDiv; } </script> <style> .btn-file { position: relative; overflow: hidden; } .btn-file input[type=file] { position: absolute; top: 0; right: 0; min-width: 100%; min-height: 100%; font-size: 100px; text-align: right; filter: alpha(opacity=0); opacity: 0; outline: none; background: white; cursor: inherit; display: block; } </style> </head> <body> <div class="container"> <div class="header"> <nav> <ul class="nav nav-pills pull-right"> <li role="presentation" class="active"><a href="#">Dasboard</a></li> <li role="presentation"><a href="/userHome">My List</a></li> <li role="presentation"><a href="/showAddBlog">Add Item</a></li> <li role="presentation" ><a href="/logout">Logout</a></li> </ul> </nav> <img src="/static/images/Flask_Icon.png" alt="Flask_Icon.png"/ > </div> <div class="well"> <footer class="footer"> <p>©etaman.com 2017</p> </footer> </div> </div> </body> </html>
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization