7. Hello World - MVC
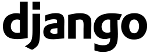
It seems that there are some debates on the role of the Django's view in MVC's perspective. However, in general, Django adheres to the MVC framework.
The three pieces, data access logic, business logic, and presentation logic are the components called the Model-View-Controller (MVC) pattern of software design. In this pattern, Model refers to the data access layer, View refers to the part of the system that selects what to display and how to display it, and Controller refers to the part of the system that decides which view to use, depending on user input, accessing the model as needed.
Note that in Django the View not only displays but also decides what to display, which is a little bit different from other MVC used in Ruby on Rails and other frameworks.
Here is the brief description on MVC:
- M: the data-access portion, is handled by Django's database layer.
- V: the portion that selects which data to display and how to display it, is handled by views and templates.
- C: the portion that delegates to a view depending on user input, is handled by the framework itself by following our URLconf and calling the appropriate Python function for the given URL.
HelloWorldApp/models.py:
from django.db import models class Line(models.Model): # model - class - table text = models.CharField(max_length=255) # field - instance - row
Here is the view we used in HelloWorldApp/views.py:
from django.shortcuts import render_to_response from models import Line def foo(request,): return render_to_response("helloDJ/home.html", {"lines" : Line.objects.all()})
Actually, template comes in here and intermediates the view regarding "how to display ('lines')". In Django, the View works as the bridge between models and templates. In other words, view takes in HTTP requests, interact with the models and then pass the models onto the templates.
Note that the code also selects which one to display, here "Line.objects.all()"
Without MVC's help, it could look like this:
from django.shortcuts import render import MySQLdb def foo(request): db = MySQLdb.connect(user='me', db='mydb', passwd='secret', host='localhost') cursor = db.cursor() cursor.execute('SELECT line FROM Line') names = [row[0] for row in cursor.fetchall()] db.close() return render(request, 'helloDJ/home.html', {'lines': names})
With this kind of coupled code, we see the issues immediately:
- The hard-coded the database connection parameters.
- The tie to MySQL.
Via Django's database layer, we separate model from view.
Here is the url configuration we used in HelloWorld/urls.py:
from django.conf.urls import patterns, include, url from HelloWorldApp.views import foo #from django.contrib import admin #admin.autodiscover() urlpatterns = patterns('', # Examples: # url(r'^blog/', include('blog.urls')), # url(r'^admin/', include(admin.site.urls)), url(r'^$', foo, name='home'), url(r'HelloWorldApp/$', foo), )
The controller decides which view to use (HelloWorldApp.views), depending on user input. Also, it is calling the appropriate Python function (foo()) for the given URL.
Sometimes, MVC in Django called MTV(models, templates and views).
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization