14. Blog app - syncdb B
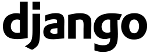
In previous chapter, we checked the SQL schema via SQLite Database Browser. Now, it's time to fill the data. We can do it through Django, but we may get around it.
Before filling in data, let's check what are the actual commands for making sql schema:
$ python manage.py sql blog BEGIN; CREATE TABLE "blog_blog" ( "id" integer NOT NULL PRIMARY KEY, "title" varchar(250) NOT NULL, "body" text NOT NULL, "publish_date" datetime NOT NULL, "gPlus" integer NOT NULL ) ; COMMIT;
Here is our models.py we wrote while ago:
from django.db import models # Create your models here. class Blog(models.Model): title = models.CharField(max_length=250) body = models.TextField() publish_date = models.DateTimeField('published date') gPlus = models.IntegerField()
Let's filling in the blog table using python shell:
$ python manage.py shell Python 2.7.5+ (default, Feb 27 2014, 19:37:08) [GCC 4.8.1] on linux2 Type "help", "copyright", "credits" or "license" for more information. (InteractiveConsole) >>> from blog.models import Blog >>> Blog.objects.all() [] >>>
We see no objects of Blog. So, we need to make an instance of it. Note that we need time zone info, we need to import timezone:
>>> from django.utils import timezone >>> b = Blog(title="blog 1", body="test blog 1", publish_date=timezone.now(), gPlus=0) >>> b <Blog: Blog object>
This created an instance of the Blog class. To save it, we issue save():
>>> b.save()
Also, we can check id:
>>> b.id 1
Let's create more:
>>> b = Blog(title="blog 2", body="test blog 2", publish_date=timezone.now(), gPlus=0) >>> b.save() >>> b = Blog(title="blog 3", body="test blog 3", publish_date=timezone.now(), gPlus=0) >>> b.save() >>> >>> Blog.objects.all() [<Blog: Blog object>, <Blog: Blog object>, <Blog: Blog object>] >>>
We can see we have 3 objects now. However, we can't tell which is which. So, what should we do about it?
To differentiate each instance, we need to put __unicode__() into our models.py:
from django.db import models # Create your models here. class Blog(models.Model): title = models.CharField(max_length=250) body = models.TextField() publish_date = models.DateTimeField('published date') gPlus = models.IntegerField() def __unicode__(self): return self.title
Since Django's model base class automatically derives __str__() from __unicode__(), we can replace the Blog object in <Blog: Blog object> with title.
Let's go back to python shell:
$ python manage.py shell Python 2.7.5+ (default, Feb 27 2014, 19:37:08) [GCC 4.8.1] on linux2 Type "help", "copyright", "credits" or "license" for more information. (InteractiveConsole) >>> from blog.models import Blog >>> Blog.objects.all() [<Blog: blog 1>, <Blog: blog 2>, <Blog: blog 3>] >>>
Now we can tell which is which!
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization