11. Django 1.8 Server Build - CentOS 7 hosted on VPS - TinyMCE
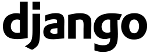
TinyMCE is a platform-independent web-based JavaScript/HTML WYSIWYG editor control software under. It has the ability to convert HTML text area fields or other HTML elements to editor instances. TinyMCE is designed to easily integrate with content management systems, including Django, Drupal, Joomla!, WordPress and SOY CMS.
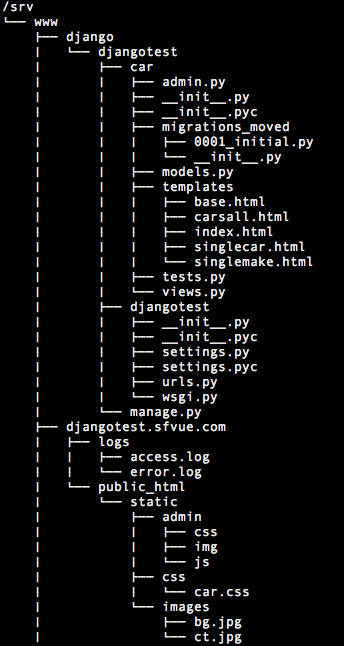
To install django-tinymce-1.5.3:
$ sudo pip install django-tinymce Downloading/unpacking django-tinymce Downloading django-tinymce-1.5.3.tar.gz (2.4MB): 2.4MB downloaded Running setup.py (path:/tmp/pip-build-Y0N_Mx/django-tinymce/setup.py) egg_info for package django-tinymce ... Successfully installed django-tinymce Cleaning up...
It's installed in /usr/lib/python2.7/site-packages/tinymce:
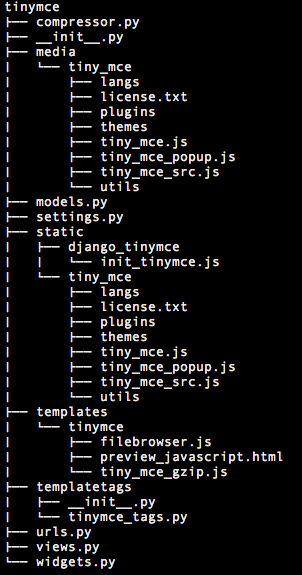
We want to add tinyMCE to INSTALLED_APPS in settings.py:
INSTALLED_APPS = ( 'django.contrib.admin', 'django.contrib.auth', 'django.contrib.contenttypes', 'django.contrib.sessions', 'django.contrib.messages', 'django.contrib.staticfiles', 'car', 'tinymce', )
Let's make database table for tinyMCE to work:
$ python manage.py syncdb /usr/lib64/python2.7/site-packages/django/core/management/commands/syncdb.py:24: RemovedInDjango19Warning: The syncdb command will be removed in Django 1.9 warnings.warn("The syncdb command will be removed in Django 1.9", RemovedInDjango19Warning) Operations to perform: Synchronize unmigrated apps: staticfiles, car, tinymce, messages Apply all migrations: admin, contenttypes, auth, sessions Synchronizing apps without migrations: Creating tables... Running deferred SQL... Installing custom SQL... Running migrations: No migrations to apply.
Now we want to add a hookup for tinyMCE in urls.py:
from django.conf.urls import include, url from django.contrib import admin urlpatterns = [ url(r'^admin/', include(admin.site.urls)), url(r'^tinymce/', include('tinymce.urls')), url(r'^$', 'car.views.index'), url(r'^cars/$', 'car.views.CarsAll'), url(r'^cars/(?P<carslug>.*)/$', 'car.views.SpecificCar'), url(r'^makes/(?P<makeslug>.*)/$', 'car.views.SpecificMake'), ]
In that way, we make it more clean. Actually, we can look what's in the tinymce.urls:
try: from django.conf.urls import url, patterns except: from django.conf.urls.defaults import url, patterns urlpatterns = patterns('tinymce.views', url(r'^js/textareas/(?P<name>.+)/$', 'textareas_js', name='tinymce-js'), url(r'^js/textareas/(?P<name>.+)/(?P<lang>.*)$', 'textareas_js', name='tinymce-js-lang'), url(r'^spellchecker/$', 'spell_check'), url(r'^flatpages_link_list/$', 'flatpages_link_list'), url(r'^compressor/$', 'compressor', name='tinymce-compressor'), url(r'^filebrowser/$', 'filebrowser', name='tinymce-filebrowser'), url(r'^preview/(?P<name>.+)/$', 'preview', name='tinymce-preview'), )
First, we want to make a js directory under static:
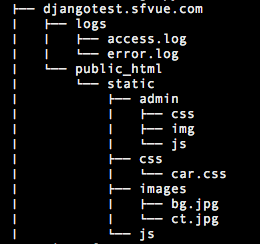
Now, copy the tinymce/media/tiny_mce directory user static/js:
$ cp -r /usr/lib/python2.7/site-packages/tinymce/media/tiny_mce /srv/www/djangotest.sfvue.com/public_html/static/js
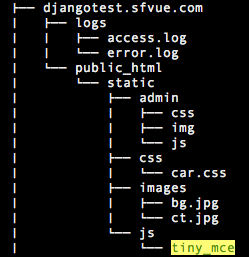
$ pwd /srv/www/djangotest.sfvue.com/public_html/static/js/tiny_mce $ ls langs license.txt plugins themes tiny_mce.js tiny_mce_popup.js tiny_mce_src.js utils
Let's make a new application that provides CMS functionality.
$ python manage.py startapp pages
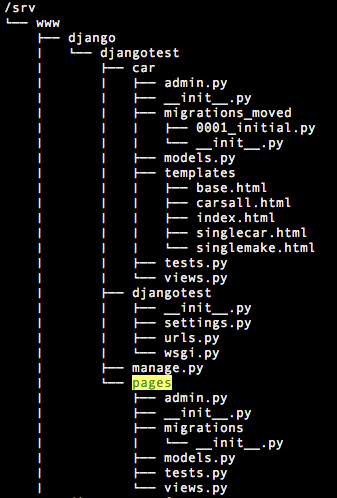
$ cd pages $ ls admin.py __init__.py migrations models.py tests.py views.py
from django.db import models class HomePage(models.Model): homecopy = models.TextField() def __unicode__(self): return 'Home Page Copy'
from django.contrib import admin from pages.models import HomePage admin.site.register(HomePage)
Since we've just created pages app, we need to modify settings.py:
INSTALLED_APPS = ( 'django.contrib.admin', 'django.contrib.auth', 'django.contrib.contenttypes', 'django.contrib.sessions', 'django.contrib.messages', 'django.contrib.staticfiles', 'car', 'tinymce', 'pages', )
Let's update db for pages:
$ cd pages $ ls admin.py __init__.py migrations models.pyc views.py admin.pyc __init__.pyc models.py tests.py $ mv migrations migrations-moved $ cd .. $ python manage.py syncdb /usr/lib64/python2.7/site-packages/django/core/management/commands/syncdb.py:24: RemovedInDjango19Warning: The syncdb command will be removed in Django 1.9 warnings.warn("The syncdb command will be removed in Django 1.9", RemovedInDjango19Warning) Operations to perform: Synchronize unmigrated apps: staticfiles, car, tinymce, messages, pages Apply all migrations: admin, contenttypes, auth, sessions Synchronizing apps without migrations: Creating tables... Creating table pages_homepage Running deferred SQL... Installing custom SQL... Running migrations: No migrations to apply.
Now, we have Home pages in our admin page:
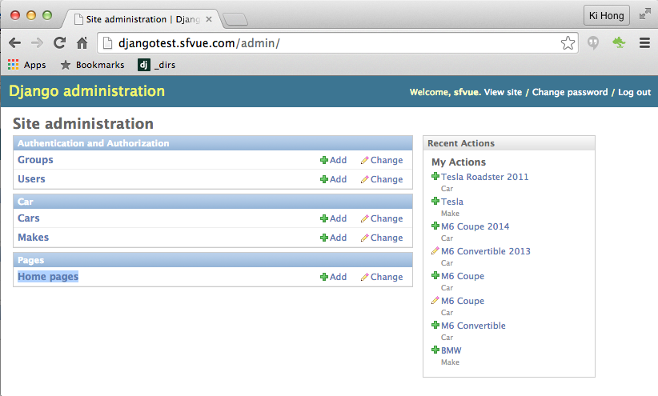
On click "Home pages":
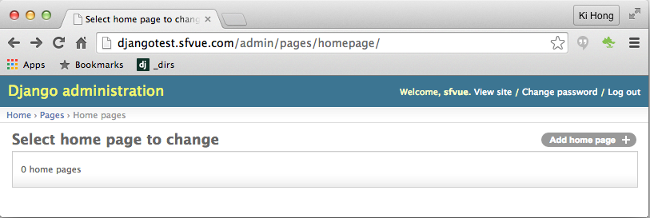
If we click "Add home page+", we get the following page with text field:
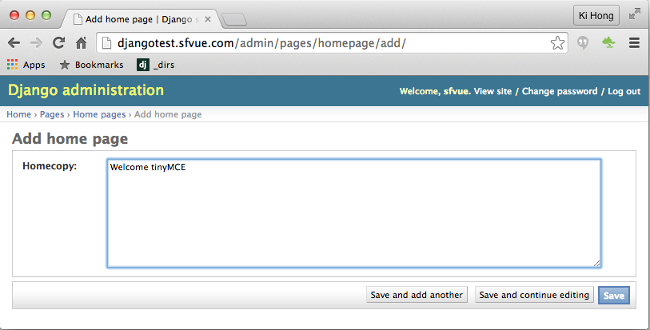
Press "Save" button:
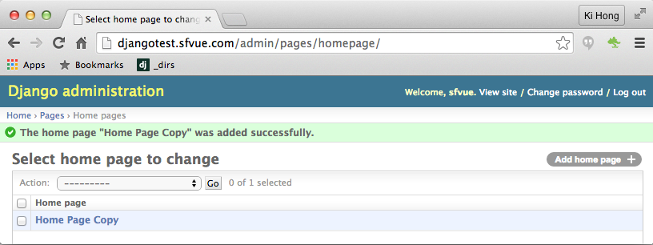
from django.shortcuts import render_to_response from django.template import RequestContext from pages.models import HomePage def MainHomePage(request): homepage = HomePage.objects.get(pk=1) context = {'homepage': homepage} return render_to_response('index.html', context, context_instance=RequestContext(request))
from django.conf.urls import include, url from django.contrib import admin urlpatterns = [ url(r'^admin/', include(admin.site.urls)), url(r'^tinymce/', include('tinymce.urls')), url(r'^$', 'pages.views.HomePage'), url(r'^cars/$', 'car.views.CarsAll'), url(r'^cars/(?P<carslug>.*)/$', 'car.views.SpecificCar'), url(r'^makes/(?P<makeslug>.*)/$', 'car.views.SpecificMake'), ]
Now, let's restart our server:
$ sudo apachectl restart
But still our home page stays the same:
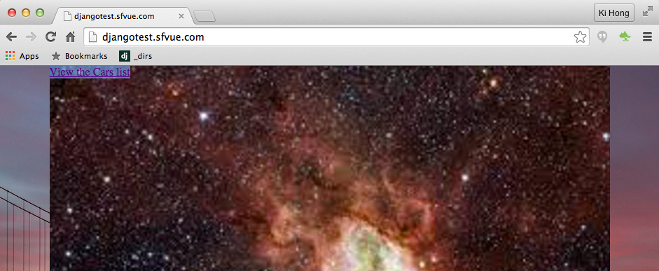
As we can see from the code (pages/views.py below, now the variable homepage is passed to our djangotest/car/templates/index.html. So, index.html can have access to variable homepage:
def MainHomePage(request): homepage = HomePage.objects.get(pk=1) context = {'homepage': homepage} return render_to_response('index.html', context, context_instance=RequestContext(request))
pages/models.py tells us that homecopy is the field variable we're looking for:
from django.db import models class HomePage(models.Model): homecopy = models.TextField() def __unicode__(self): return 'Home Page Copy'
We're adding another line to the page:
{% extends "base.html" %} {% block content %} <div id="home_copy"> {{ homepage.homecopy }} </div> <a href="cars/">View the Cars list</a> {% endblock %}
Now, if we refresh the page, we get a new one:
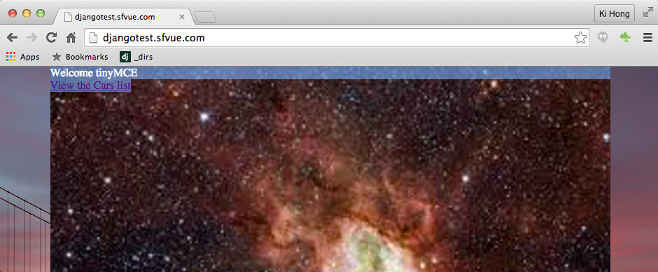
Continued in 12. TinyMCE 2
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization