9. Django 1.8 Server Build - CentOS 7 hosted on VPS - Views (templates and css)
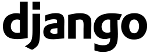
In previous chapter (8. Django 1.8 Server Build - CentOS 7 hosted on VPS - Model 3 (using shell)), we learned how to interface with all the objects that are stored in our models.
The materials we learned in the previous chapter will be the basis of linking models to views.
In this chapter, we'll use shell to update our models and views.
We'll use two images, and put them under static folder:
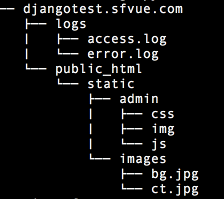
Also, we need a directory called templates under our app car folder:
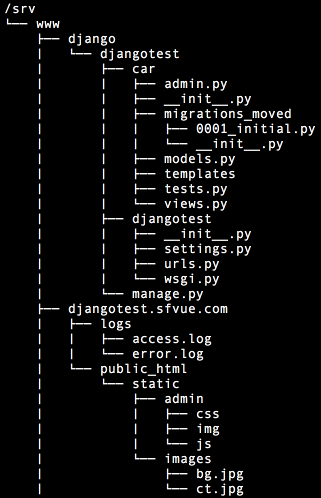
Our project's TEMPLATES setting describes how Django will load and render templates. The default settings file configures a DjangoTemplates backend whose APP_DIRS option is set to True.
TEMPLATES = [ { 'BACKEND': 'django.template.backends.django.DjangoTemplates', 'DIRS': [], 'APP_DIRS': True, 'OPTIONS': { 'context_processors': [ 'django.template.context_processors.debug', 'django.template.context_processors.request', 'django.contrib.auth.context_processors.auth', 'django.contrib.messages.context_processors.messages', ], }, }, ]
By convention DjangoTemplates looks for a templates subdirectory in each of the INSTALLED_APPS.
Note that the root directory djangotest/ (under django directory) is just a project container, and we can rename it anything we like.
The inner djangotest/ directory is the actual Python package for our project.
The car is our app, and this directory structure will house the car application.
from django.shortcuts import render_to_response from django.template import RequestContext from car.models import Car def CarsAll(request): cars = Car.objects.all().order_by('name') context = {'cars': cars} return render_to_response('carsall.html',context, context_instance=RequestContext(request))
The package django.shortcuts collects helper functions and classes that "span" multiple levels of MVC. In other words, these functions/classes introduce controlled coupling for convenience's sake.
note that we're importing Car class from models.py of car app.
The render_to_response(() renders a given template with a given context dictionary and returns an HttpResponse object with that rendered text.
What we've done here?
We get some information from our database:
cars = Car.objects.all().order_by('name')
Then, we pass the info to our template which will be defined soon. Also, we render it:
return render_to_response('carsall.html',context, context_instance=RequestContext(request))
Now that our view is using barsall.html template, we may want to define it. In the next section, we'll define the base.html first, and then will work on the barsall.html template.
car/templates/base.html, defines a simple HTML skeleton document.
<html> <head> <link rel="stylesheet" type="text/css" href="/static/css/car.css" /> {% block extrahead %}{% endblock %} </head> <body> <div id="pageContainer"> {% block content %} {% endblock %} </div> </body> </html>
We used tags, and it looks like this: {% tag %}. Tags are more complex than variables: Some create text in the output, some control flow by performing loops or logic, and some load external information into the template to be used by later variables.
Some tags such as block require beginning and ending tags
(i.e. {% tag %} ... tag contents ... {% endtag %})
Remember the carsall.html is called from base.html, so we need to work on it.
It looks like this:
{% extends "base.html" %} {% block content %} <div id="carslist"> {% for car in cars %} {{ car }} {% endfor %} </div>> {% endblock %}
The extends tag is the key here. It tells the template engine that this template "extends" another template. When the template system evaluates this template, first it locates the parent - in this case, base.html.
At that point, the template engine will notice the tags in base.html and replace those blocks with the contents of the child template.
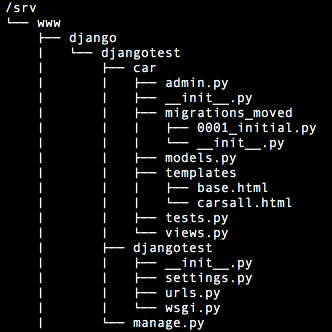
In the previous section, we defined CarsAll() in car/views.py, and we want to put it into our urls.py in car app:
from django.conf.urls import include, url from django.contrib import admin urlpatterns = [ url(r'^admin/', include(admin.site.urls)), url(r'^cars/$', 'car.views.CarsAll'), ]
Let's restart our web server:
$ sudo apachectl restart
From the work so far, we get the following list of cars in order:
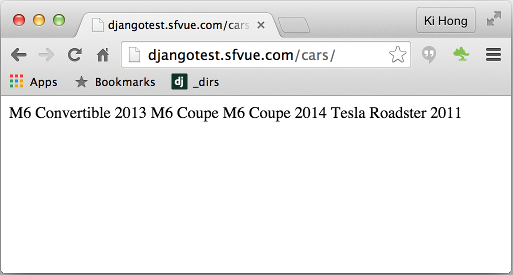
Actually, the output is the alphabetic order of the following page:
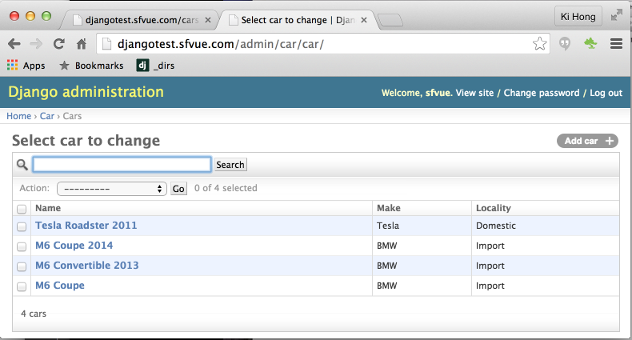
Now we want to work on /srv/www/djangotest.sfvue.com/public_html/static/css/car.css:
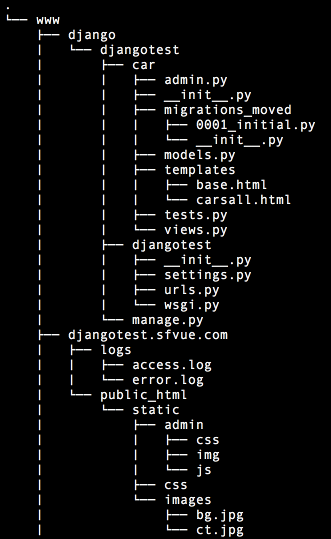
We'll add a background image (/static/images/bg.jpg) to the page:
body { background-attachment: fixed; background-color: #000000; background-image: url(/static/images/bg.jpg); background-position:center top; background-repeat: no-respect; color: #FFFFFF; margin: 0; padding: 0; }
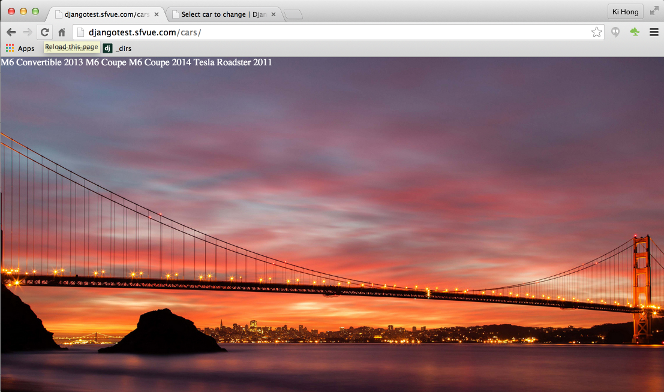
We want to add a center image:
body { background-attachment: fixed; background-color: #000000; background-image: url(/static/images/bg.jpg); background-position:center top; background-repeat: no-respect; color: #FFFFFF; margin: 0; padding: 0; } #pageContainer { background: url(/static/images/ct.jpg) repeat-y scroll center top #000000; margin: 0 auto; position: relative; width: 800px; min-height: 800px; }
Here is our new page with the added image:
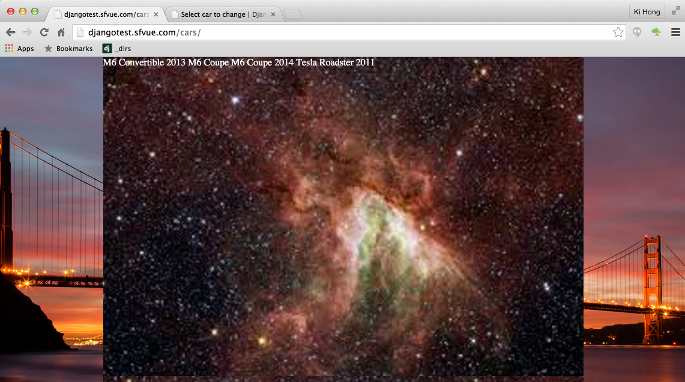
Continued in 10. Views 2 (home page and more templates)
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization