7. Django 1.8 Server Build - CentOS 7 hosted on VPS - Model 2
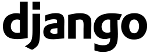
In previous chapter (6. Django 1.8 Server Build - CentOS 7 hosted on VPS - Model), we setup Django model.
In this chapter, we'll populate the tables Cars and Makes.
Let's start filling the data for Cars:
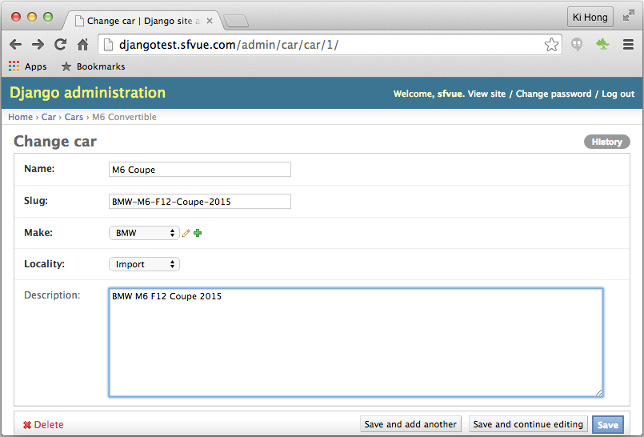
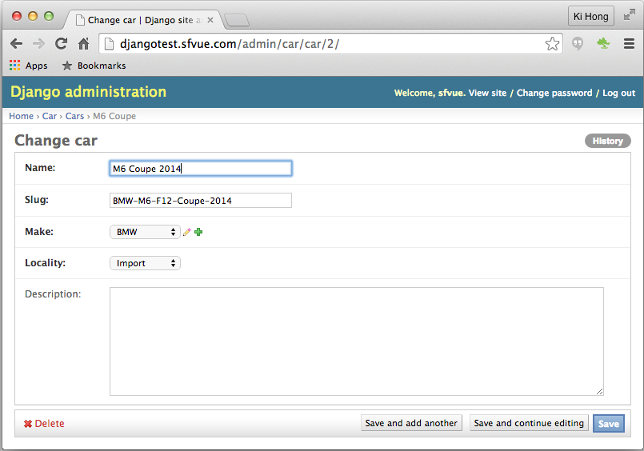
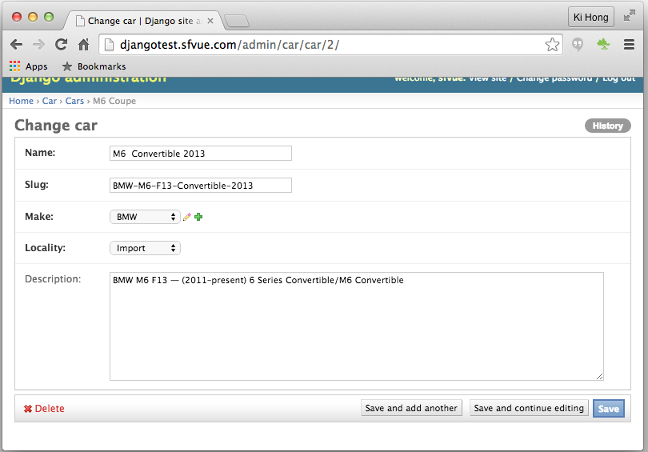
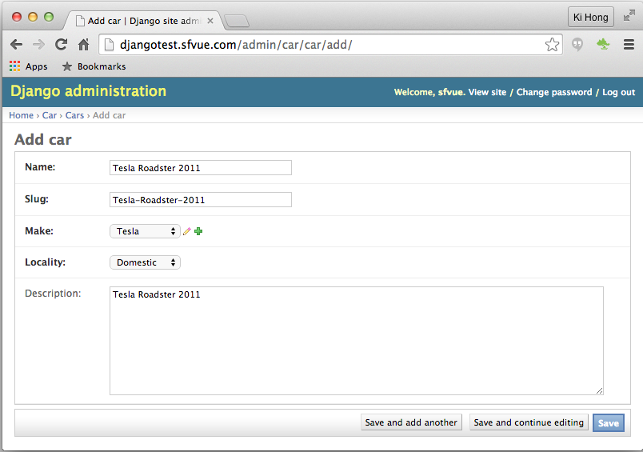
Here is the table we built so far.
Cars:
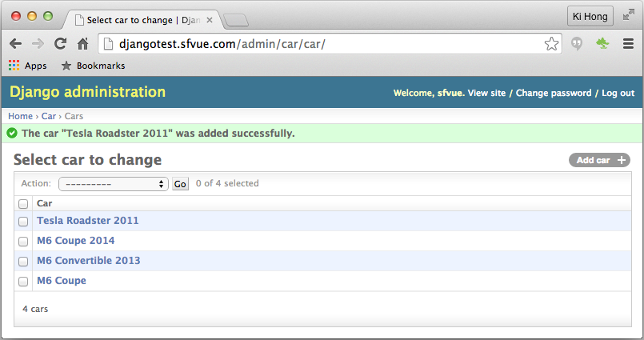
And Makes:
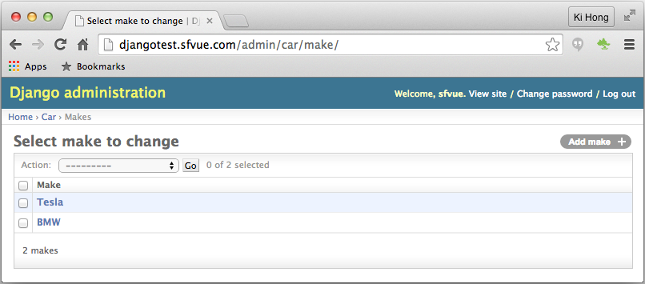
Note that the name is the returned value from __unicode__(self):
class Car(models.Model): name = models.CharField(max_length=200) slug = models.SlugField(unique=True) make = models.ForeignKey('Make') locality = models.CharField(max_length=1, choices=CAR_CHOICES) description = models.TextField(blank=True) def __unicode__(self): return self.name class Make(models.Model): name = models.CharField(max_length=200) slug = models.SlugField(unique=True) description = models.TextField(blank=True) def __unicode__(self): return self.name
We want to the Slug field can be filled automatically when we type the name.
Let's go to the car app, and edit admin.py:
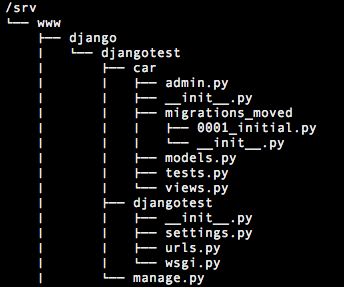
from django.contrib import admin from car.models import Car, Make class CarAdmin(admin.ModelAdmin): prepopulated_fields = {'slug':('name',)} admin.site.register(Car, CarAdmin) admin.site.register(Make)
Restart the web server:
$ sudo apachectl restart
As we can see the picture below, while we typing the name of a car, the slug is filled automatically:
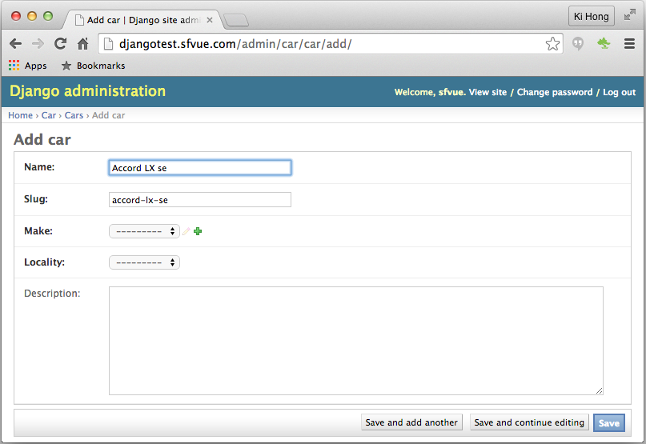
We can do the same to Make as well:
from django.contrib import admin from car.models import Car, Make class CarAdmin(admin.ModelAdmin): prepopulated_fields = {'slug':('name',)} list_display = ('name','make','locality') class MakeAdmin(admin.ModelAdmin): prepopulated_fields = {'slug':('name',)} admin.site.register(Car, CarAdmin) admin.site.register(Make, MakeAdmin)
Note that we also add the list_display.
Restart the server:
$ sudo apachectl restart
Refresh the Admin page:
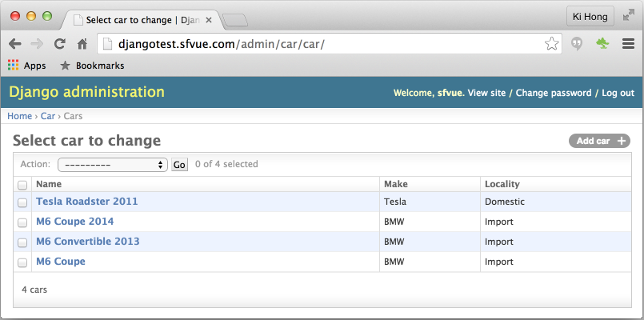
Aw we can see from the picture, thanks to the list_display, we now have additional two columns.
If we click "Make", it will have sorted display:
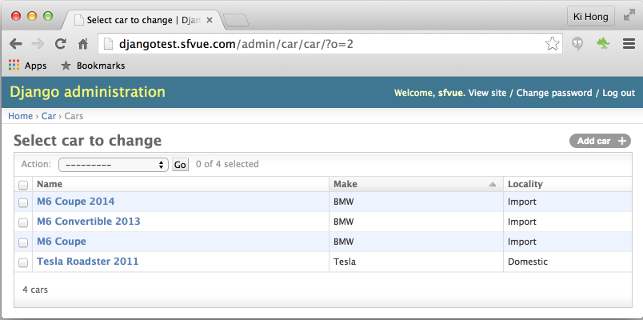
Now we want to add "search":
from django.contrib import admin from car.models import Car, Make class CarAdmin(admin.ModelAdmin): prepopulated_fields = {'slug':('name',)} list_display = ('name','make','locality') search_field = ['name'] class MakeAdmin(admin.ModelAdmin): prepopulated_fields = {'slug':('name',)} admin.site.register(Car, CarAdmin) admin.site.register(Make, MakeAdmin)
Restart the server again:
$ sudo apachectl restart
Refresh the Admin page:
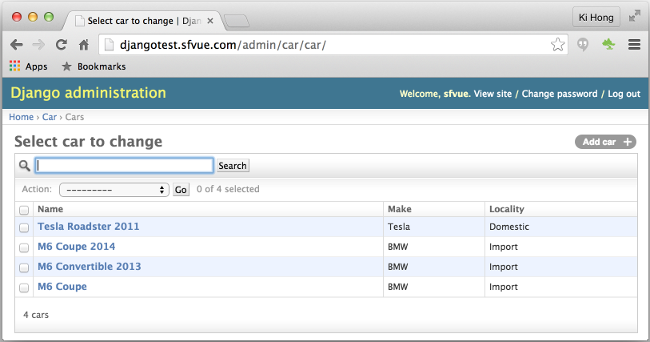
Continued in 8. Model 3 (using shell)
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization