HTML5 Tutorial: Forms - 2020
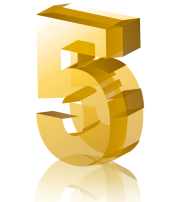
Web application developers find themselves continually in need of some more sophisticated form controls, such as spinners, sliders, date/time pickers, color pickers, and so on.
In order to tap into these types of controls, developers needed to use an external JavaScript library that provided UI components, or else use an alternative development framework such as Adobe Flex, Microsoft Silverlight, or JavaFX.
HTML5 aims to fill some of the gaps left by its predecessor in this space by providing a whole range of new form input types. Search boxes, text inputs, and other fields get better controls for focusing, validating data, interacting with other page elements, sending through email, and more.
In addition to these new input types, HTML5 also supports two major new features for form fields. The first of these is the placeholder attribute, which allows the developer to define the text that will appear in a textbox-based control when its contents are empty. An example of this would be a search box where the developer would prefer not to use a label outside the box itself. The placeholder attribute allows the developer to specify text that will show when the value of the control is empty and the control does not have focus. The second enhancement is autofocus, which tells a browser to automatically give focus to a particular form field when the page has rendered, without requiring JavaScript code to do so.
The first improvement HTML5 brings to web forms is the ability to set placeholder text in an input field. Placeholder text is displayed inside the input field as long as the field is empty and not focused. As soon as we click on or tab to the input field, the placeholder text disappears.
Here's how we can include placeholder text in our own web forms:
<form> <input name="myInput" placeholder="Search Bookmarks and History"> <input type="submit" value="Search"> </form>
Browsers that don't support the placeholder attribute will simply ignore it.
Many web sites use JavaScript to focus the first input field of a web form automatically. While this is convenient for most people, it can be annoying for power users or people with special needs. If we press the space bar expecting to scroll the page, the page will not scroll because the focus is already in a form input field. (It types a space in the field instead of scrolling.) If we focus a different input field while the page is still loading, the site's autofocus script may move the focus back to the original input field, disrupting our flow and causing us to type in the wrong place.
Because the autofocusing is done with JavaScript, it can be tricky to handle all of these edge cases, and there is little recourse for people who don't want a web page to steal the focus.
To solve this problem, HTML5 introduces an autofocus attribute on all web form controls. The autofocus attribute does exactly what it says on the tin: as soon as the page loads, it moves the input focus to a particular input field. But because it's just markup instead of script, the behavior will be consistent across all web sites.
Example of setting a form field to autofocus:
<form> <input name="myName" autofocus> <input type="submit" value="Search"> </form>
As it happens to the placeholder, browsers that don't support the autofocus attribute will simply ignore it. If we want our autofocus fields to work in all browsers, not just these HTML5 browsers, we can keep our current autofocus script with just two small changes:
- Add the autofocus attribute to our HTML markup
- Detect if the browser supports the autofocus attribute, and only run our own autofocus script if the browser doesn't support autofocus natively.
<form name="myForm"> <input id="myInput" autofocus> <script> if (!("autofocus" in document.createElement("input"))) { document.getElementById("myInput").focus(); } </script> <input type="submit" value="Go"> </form>
The most common web forms that we've been using are:
Field Type | HTML | Note |
---|---|---|
checkbox | <input type="checkbox"> | can be toggled on or off |
radio button | <input type="radio"> | can be grouped with other inputs |
password field | <input type="password"> | echoes dots instead of characters as we type |
drop-down lists | <select> <option>... | |
file picker | <input type="file> | pops up an open file dialog |
submit button | <input type="submit"> | |
plain text | <input type="text"> | the type attribute can be omitted |
All of these input types still work in HTML5. If we upgrade to HTML5, we don't need to make a single change to our web forms. However, HTML5 defines several new field types, and for reasons that will become clear in a moment, there is no reason not to start using them. The first of these new input types is for email addresses. It looks like this:
<form> <input type="email"> <input type="submit" value="Go"> </form>
All browsers support type="email". They may not do anything special with it, but browsers that don't recognize type="email" will treat it as type="text" and render it as a plain text field.
The web has millions of forms that ask us to enter an email address, and all of them use <input type="text">. We see a text box, we type our email address in the text box. Along comes HTML5, which defines type="email". Do browsers freak out? No. Every single browser on Earth treats an unknown type attribute as type="text" - even IE 6. So we can upgrade our web forms to use type="email" right now.
The HTML5 specification doesn't mandate any particular user interface for the new input types. Opera styles the form field with a small email icon. Other HTML5 browsers like Safari and Chrome simply render it as a text box - exactly like type="text" - so our users will never know the difference (unless they view-source).
URLs are another type of specialized text. The syntax of a web address is constrained by the relevant Internet standards. If someone asks us to enter a web address into a form, they're expecting something like "//www.google.com/", not "125 Farwood Road." Forward slashes are common - even Google's home page has three of them. Periods are also common, but spaces are forbidden. And every web address has a domain suffix like .com or .org.
<input type="url">
Browsers that don't support HTML5 will treat type="url" exactly like type="text", so there's no downside to using it for all our web-address-inputting needs.
Pick a number. A number in a particular range. We may only want certain kinds of numbers within that range - maybe whole numbers but not fractions or decimals, or something more esoteric like numbers divisible by 10. HTML5 has we covered.
<input type="number" min="0" max="10" step="2" value="6">
Here is how it looks like:
Opera respects the HTML 5 spinbox, and it looks like this:
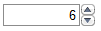
Let's take that one attribute at a time.
- type="number" means that this is a number field.
- min="0" specifies the minimum acceptable value for this field.
- max="10" is the maximum acceptable value.
- step="2", combined with the min value, defines the acceptable numbers in the range: 0, 2, 4, and so on, up to the max value.
- value="6" is the default value. This should look familiar. It's the same attribute name we've always used to specify values for form fields.
That's the markup side of a number field. Keep in mind that all of those attributes are optional. If we have a minimum but no maximum, we can specify a min attribute but no max attribute. The default step value is 1, and we can omit the step attribute unless we need a different step value. If there's no default value, then the value attribute can be blank or even omitted altogether.
But HTML5 doesn't stop there. For the same low, low price of free, we get these handy JavaScript methods as well:
- input.stepUp(n) increases the field's value by n.
- input.stepDown(n) decreases the field's value by n.
- input.valueAsNumber returns the current value as a floating point number. (The input.value property is always a string.)
As with all the other input types, browsers that don't support type="number" will treat it as type="text". The default value will show up in the field (since it's stored in the value attribute), but the other attributes like min and max will be ignored. We're free to implement them ourselves, or we could reuse one of the many JavaScript frameworks that have already implemented spinbox controls. Just check for the native HTML5 support first, like this:
if (!Modernizr.inputtypes.number) { // no native support for type=number fields // maybe try Dojo or some other JavaScript framework }
Modernizr is an open source, MIT-licensed JavaScript library that detects support for many HTML5 & CSS3 features. We should always use the latest version. To use it, include the following <script>element at the top of our page.
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>HTML 5</title> <script src="modernizr-1.6.min.js"></script> </head> <body> ... </body> </html>
Modernizr runs automatically. There is no modernizr_init() function to call. When it runs, it creates a global object called Modernizr, which contains a set of Boolean properties for each feature it can detect. For example, if our browser supports the canvas API, the Modernizr.canvas property will be true. If our browser does not support the canvas API, the Modernizr.canvas property will be false.
if (Modernizr.canvas) { // let's draw some shapes! } else { // no native canvas support available :( }
Check how does slider controls look like in our browser:
The look may be different depending on the browsers.
IE, FireFox | Opera | Chrome |
---|---|---|
![]() |
![]() |
![]() |
We can now have slider controls in our web forms, too. The markup looks similar to spinbox.
<input type="range" min="0" max="10" step="2" value="6">
All the available attributes are the same as type="number", min, max, step, value, and they mean the same thing. The only difference is the user interface. Instead of a field for typing, browsers are expected to render type="range" as a slider control. At time of writing, the latest versions of Safari, Chrome, and Opera all do this. All other browsers simply treat the field as type="text", so there's no reason we can't start using it immediately.
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization