Javascript : text input and event listener
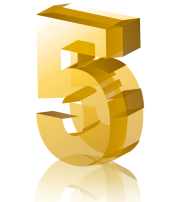
bogotobogo.com site search:
Text input
<html> <head> <meta charset="utf-8"> <title>HTML Starter</title> </head> <body> Name: <input type="text"/> <br /> <br /> Hello! </body> </html>
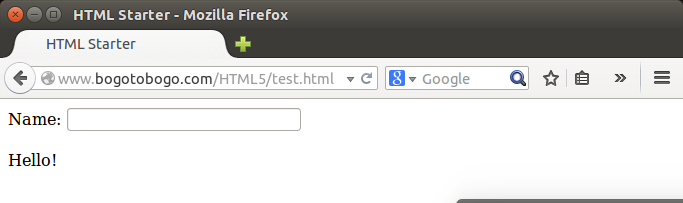
bogotobogo.com site search:
Keyup event listener on textInput
<html> <head> <meta charset="utf-8"> <title>HTML Starter</title> </head> <body> Name:<input id="textInput" type="text"/> <br /> <br /> Hello! <script> var textInputElement = document.getElementById('textInput'); textInputElement.addEventListener('keyup', function(){ console.log('Got keyup event.'); }); </script> </body> </html>
The console.log is used for debugging. We can log some-event to the console when something happens. For instance:
$( '#someButton' ).click ( function () { console.log ( '#someButton was clicked' ); // do something } );
We then see #someButton was clicked in Firebug (or Chrome)'s "Console" tab when we click the button. In our html, it's keyup event as shown in the Chrome:
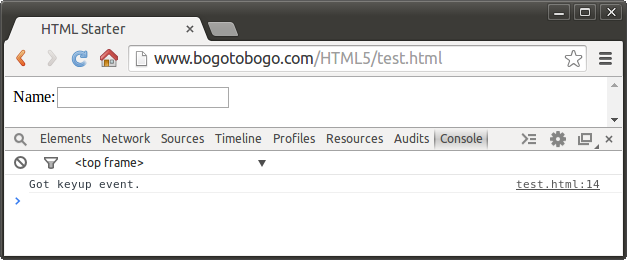
Extracting text from the text input as it changes
<html> <head> <meta charset="utf-8"> <title>HTML Starter</title> </head> <body> Name:<input id="textInput" type="text"/> <br /> <br /> Hello! <script> var textInputElement = document.getElementById('textInput'); textInputElement.addEventListener('keyup', function(){ var text = textInputElement.value; console.log('New text is "' + text + '"'); }); </script> </body> </html>
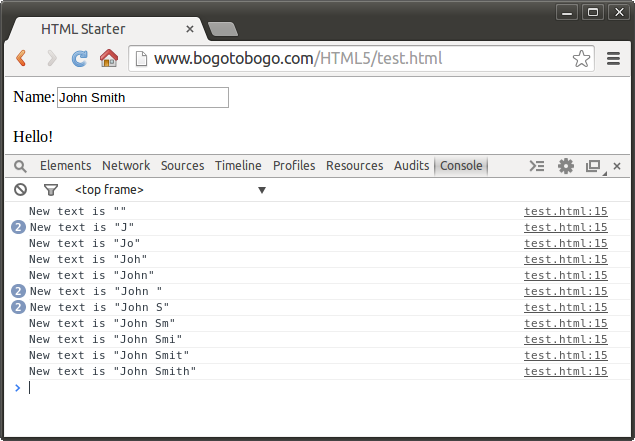
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization