Jenkins on EC2 : Puppet Plugin and Deployment Notification
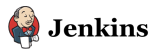
Puppet plugin builds on top of Deployment Notification Plugin and lets Puppet users track when/where/what files are deployed, and trigger other activities after deployments have happened.
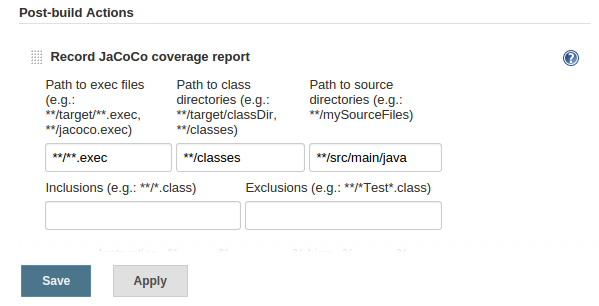
Now we want Jenkins to generate line coverage reports automatically every time it runs tests.
"Manage Jenkins"=>"Manage Plugins", then go to "Available" plugins tab, and check "JaCoCo" plugin:
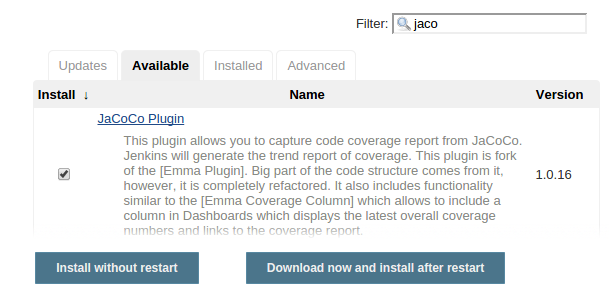
Click on "Download now and install after restart", and then check "Restart Jenkins when installation is complete..."
Let's go back to "Configuration" once Jenkins server is back on. We need to reconfigure the project to gather the JaCoCo reports and make them available through Jenkins. Under the "Post-build Actions", click on "Add post-build action", and select "Record to JaCoco coverage report".
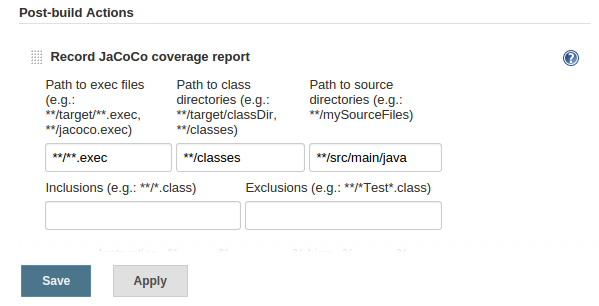
Click "Save".
Now we need to configure JaCoCo in our maven pom.xml.
Let's switch back to our repository on our local system, and edit to enable JaCoCo plugin. In other words, before the Unit test run, we need to prepare JaCoCo agent. So, we have to start JaCoCo agent, and after the Unit test run, we want to generate a report on the code coverage:
<project> <modelVersion>4.0.0</modelVersion> <groupId>com.bogotobogo</groupId> <artifactId>bogotobogo_JenkinsOnEC2</artifactId> <packaging>jar</packaging> <version>1.0-SNAPSHOT</version> <properties> <maven.compiler.source>7</maven.compiler.source> <maven.compiler.target>7</maven.compiler.target> </properties> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.11</version> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.jacoco</groupId> <artifactId>jacoco-maven-plugin</artifactId> <version>0.6.4.201312101107</version> <executions> <execution> <id>pre-unit-test</id> <goals> <goal>prepare-agent</goal> </goals> </execution> <execution> <id>post-unit-test</id> <phase>test</phase> <goals> <goal>report</goal> </goals> </execution> </executions> </plugin> </plugins> </build> </project>
BankAccount.java:
package com.bogotobogo; public class BankAccount { private double balance; public BankAccount(double balance) { this.balance = balance; } public double debit(double amount) { if (balance < amount) { amount = balance; } balance -= amount; return amount; } }
Here is the test code, TestBankAccount.java:
package com.bogotobogo; import junit.framework.Assert; import org.junit.Before; import org.junit.Test; public class TestBankAccount { @Test public void testFunds() { BankAccount account = new BankAccount(10); double amount = account.debit(5); Assert.assertEquals(5.0, amount); } }
When we work with maven project, it's better follow the standard directory layout. Here is our project layout:
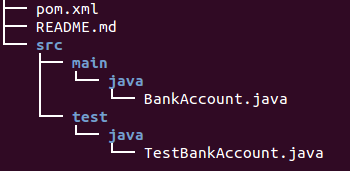
For more on Maven directory layout, visit Introduction to the Standard Directory Layout - Apache Maven Project.
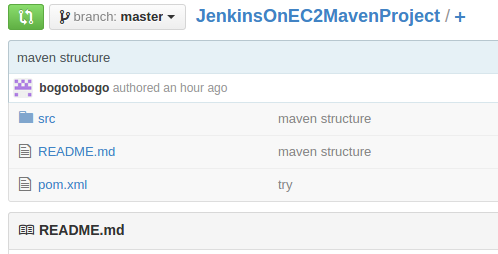
Actually, before making the Maven file structure, we got the following error:
... [INFO] skip non existing resourceDirectory /var/lib/jenkins/workspace/JenkinsOnEC2Maven/src/main/resources [INFO] [INFO] --- maven-compiler-plugin:2.0.2:compile (default-compile) @ JankinsOnEC2Maven --- [INFO] No sources to compile [INFO] [INFO] --- maven-resources-plugin:2.3:testResources (default-testResources) @ JankinsOnEC2Maven --- [WARNING] Using platform encoding (UTF-8 actually) to copy filtered resources, i.e. build is platform dependent! [INFO] skip non existing resourceDirectory /var/lib/jenkins/workspace/JenkinsOnEC2Maven/src/test/resources [INFO] [INFO] --- maven-compiler-plugin:2.0.2:testCompile (default-testCompile) @ JankinsOnEC2Maven --- [INFO] No sources to compile [INFO] [INFO] --- maven-surefire-plugin:2.10:test (default-test) @ JankinsOnEC2Maven --- [INFO] No tests to run. [INFO] Surefire report directory: /var/lib/jenkins/workspace/JenkinsOnEC2Maven/target/surefire-reports ...
But after reconstructing the structure as Maven layout, all the errors disappeared!
... ------------------------------------------------------- T E S T S ------------------------------------------------------- Running com.bogotobogo.TestBankAccount Tests run: 1, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.223 sec Results : Tests run: 1, Failures: 0, Errors: 0, Skipped: 0 [JENKINS] Recording test results [INFO] [INFO] --- jacoco-maven-plugin:0.6.4.201312101107:report (post-unit-test) @ JankinsOnEC2Maven --- [INFO] [INFO] --- maven-jar-plugin:2.2:jar (default-jar) @ JankinsOnEC2Maven --- [INFO] Building jar: /var/lib/jenkins/workspace/JenkinsOnEC2Maven/target/JankinsOnEC2Maven-1.0-SNAPSHOT.jar [INFO] [INFO] --- maven-install-plugin:2.3:install (default-install) @ JankinsOnEC2Maven --- [INFO] Installing /var/lib/jenkins/workspace/JenkinsOnEC2Maven/target/JankinsOnEC2Maven-1.0-SNAPSHOT.jar to /var/lib/jenkins/.m2/repository/com/bogotobogo/JankinsOnEC2Maven/1.0-SNAPSHOT/JankinsOnEC2Maven-1.0-SNAPSHOT.jar [INFO] Installing /var/lib/jenkins/workspace/JenkinsOnEC2Maven/pom.xml to /var/lib/jenkins/.m2/repository/com/bogotobogo/JankinsOnEC2Maven/1.0-SNAPSHOT/JankinsOnEC2Maven-1.0-SNAPSHOT.pom [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 10.107s [INFO] Finished at: Mon Nov 17 06:35:27 UTC 2014 [INFO] Final Memory: 20M/49M [INFO] ------------------------------------------------------------------------ [JENKINS] Archiving /var/lib/jenkins/workspace/JenkinsOnEC2Maven/pom.xml to com.bogotobogo/JankinsOnEC2Maven/1.0-SNAPSHOT/JankinsOnEC2Maven-1.0-SNAPSHOT.pom [JENKINS] Archiving /var/lib/jenkins/workspace/JenkinsOnEC2Maven/target/JankinsOnEC2Maven-1.0-SNAPSHOT.jar to com.bogotobogo/JankinsOnEC2Maven/1.0-SNAPSHOT/JankinsOnEC2Maven-1.0-SNAPSHOT.jar Sending e-mails to: k@bogotobogo.com channel stopped [JaCoCo plugin] Collecting JaCoCo coverage data... [JaCoCo plugin] **/**.exec;**/classes;**/src/main/java; locations are configured [JaCoCo plugin] Number of found exec files: 1 [JaCoCo plugin] Saving matched execfiles: /var/lib/jenkins/workspace/JenkinsOnEC2Maven/target/jacoco.exec [JaCoCo plugin] Saving matched class directories: /var/lib/jenkins/workspace/JenkinsOnEC2Maven/target/classes [JaCoCo plugin] Saving matched source directories: /var/lib/jenkins/workspace/JenkinsOnEC2Maven/src/main/java [JaCoCo plugin] Loading inclusions files.. [JaCoCo plugin] inclusions: [] [JaCoCo plugin] exclusions: [] [JaCoCo plugin] Thresholds: JacocoHealthReportThresholds [minClass=0, maxClass=0, minMethod=0, maxMethod=0, minLine=0, maxLine=0, minBranch=0, maxBranch=0, minInstruction=0, maxInstruction=0, minComplexity=0, maxComplexity=0] [JaCoCo plugin] Publishing the results.. [JaCoCo plugin] Loading packages.. [JaCoCo plugin] Done. Sending e-mails to: k@bogotobogo.com Finished: SUCCESS
Here is the Coverage Report from JaCoCo after running the test:
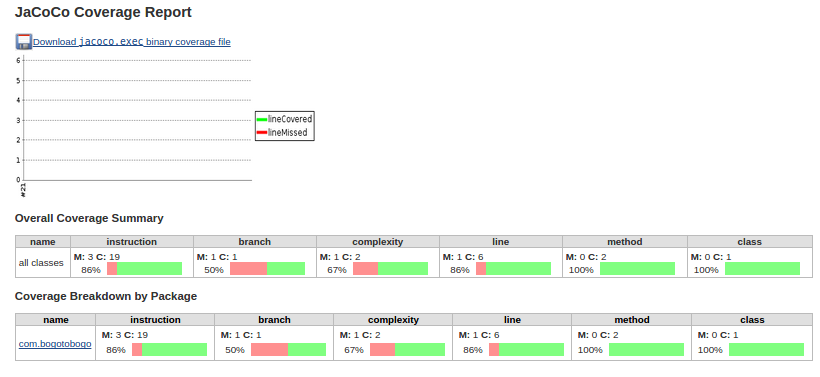
Jenkins
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization